Configuring the updater file for generated endpoints
Within the context of Cloud API, an updater file defines how information is mapped from an element resource to the corresponding data model entity. This information is used for POSTs and PATCHes.
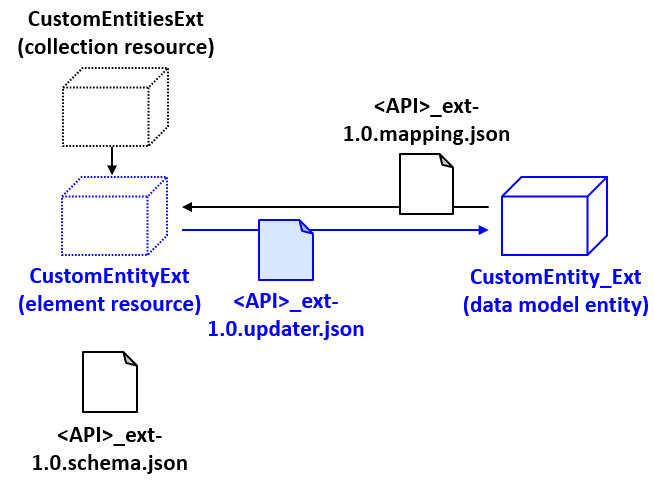
The following sections provide an overview of configuring updater files for generated endpoints. For a complete description of how to configure mapping files, see Configuring schemas.
Overview of updater file syntax
An updater file contains an updaters
section that lists one or more API
resources. For each resource, the following attributes are specified:
- The
schemaDefinition
that defines the structure of the resource- This references a schema declared in a schema.json file.
- The data model entity that serves as the
root
for mapping information for this resource. - A list of
properties
- Each property includes a
path
attribute. This defines, for each resource property, the data model entity property into which the resource field property is to be written. - Depending on the nature of the property, there may be additional attributes.
- Each property includes a
For example, the following is a portion of the updater for the base configuration
Activity
resource:
"Activity": {
"schemaDefinition": "Activity",
"root": "entity.Activity",
"properties": {
"description": {
"path": "Activity.Description"
},
"mandatory": {
"path": "Activity.Mandatory"
},
...
Note the following:
- This resource is defined in the schema who name is
Activity
(This schema is defined in some other schema.json file.) - The root for the resource mapping is
entity.Activity
. - For each instance of the resource:
- The value of the
description
property is written to the Activity entity'sDescription
field. - The value of the
mandatory
property is written to the Activity entity'sMandatory
field.
- The value of the
Note that there may be properties that appear in the mapping file but not the updater
file. This typically occurs with properties that are read-only. For example, the
Activity
entity has a closeDate
property, which
the application sets when the activity is closed. This property appears in the mapping
file, as it can be read. But it does not appear it the updater file because it cannot be
written to.
Modifications made to the updater file
The base configuration includes a set of API-specific extension updater files. The
purpose of these files is to define updater information for custom resources for a given
API. These files are named using the pattern
<API>_ext-1.0.updater.json
, where <API> is the internal name
of the API. For example, the common_ext-1.0.updater.json
file is used
to define updater information for custom resources in the Common API. You can access
extension mapping files in Studio through the integration -> mappers -> ext ->
<API>.v1 node.
When you generate endpoints for a custom entity, the REST endpoint generator adds the following code to the relevant extension updater file:
"<resourceName>": {
"schemaDefinition": "<schemaNameForResource>",
"root": "entity.<customEntity>",
// TODO RestEndpointGenerator : Add updater properties here
"properties": { }
}
CustomEntity_Ext
entity,
the following is added to the extension updater
file: "CustomEntityExt": {
"schemaDefinition": "CustomEntityExt",
"root": "entity.CustomEntity_Ext",
// TODO RestEndpointGenerator : Add updater properties here
"properties": { }
}
Note that the REST endpoint generator does not provide any properties
information. This is because the only field that gets added to the schema definition
files is the id
field. This field is not writeable, so it is not added
to the updater.
The developer is responsible for:
- Deciding which fields from the custom entity must be available for POSTs and PATCHes
- Adding updater information for those fields.
For example, suppose the CustomEntity_Ext
entity included three
fields:
CustomDescription
(a string)IsActive
(a boolean)ExpirationDate
(a datetime)
The developer decides CustomDescription
and IsActive
must be available to POSTs and PATCHes. The developer would need to add the code shown
below in bold.
"CustomEntityExt": {
"schemaDefinition": "CustomEntityExt",
"root": "entity.CustomEntity_Ext",
// TODO RestEndpointGenerator : Add updater properties here
"properties": {
"customDescription": {
"path": "CustomEntity_Ext.CustomDescription"
},
"isActive": {
"path": "CustomEntity_Ext.IsActive"
}
}
}
The developer decides ExpirationDate
must not be available to POSTs and
PATCHes. The field may appear in the schema and mapper, as it may be available for GETs.
But it is omitted from the updater as it is not available to POSTs or PATCHes.
Updater syntax for property paths
Scalars
path
property to the field in the data model entity. No other
attributes are required. For example:
"description": {
"path": "Activity.Description"
},
Compound datatypes
When you define the updater for a compound datatype (such as a
Typekey
, MonetaryAmount
,
CurrencyAmount
, or SpatialPoint
), you must
include a valueResolver
attribute with a child
typeName
attribute. This attribute specifies a resolver, which
defines how to map the structure for the compound datatype to the data model entity.
The updater syntax for compound datatypes is:
"<field>": {
"path": "<pathValue>",
"valueResolver": {
"typeName": "<resolverName>"
}
},
The following table lists the resolver names for the common compound datatypes.
Compound datatype | mapper value |
Typekey | TypeKeyValueResolver |
MonetaryAmount | MonetaryAmountValueResolver |
CurrencyAmount | CurrencyAmountValueResolver |
SpatialPoint | SpatialPointValueResolver |
Activity
resource's
assignmentStatus
field looks like
this: "assignmentStatus": {
"path": "Activity.AssignmentStatus",
"valueResolver": {
"typeName": "TypeKeyValueResolver"
}
},