Configuring the mapping file for generated endpoints
Within the context of Cloud API, a mapping file contains mappers that define how information is mapped from a data model entity to the corresponding element resource. This information is used for GETs, and for the responses of POSTs and PATCHes.
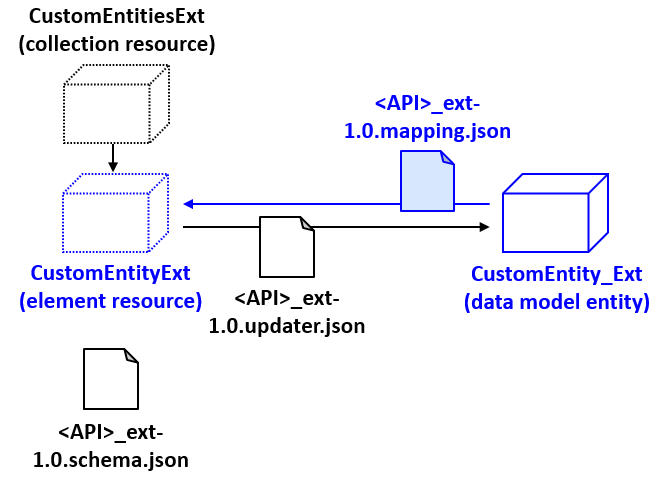
The following sections provide an overview of configuring mapping files for generated endpoints. For a complete description of how to configure mapping files, see Configuring schemas.
Overview of mapping file syntax
A mapping file contains a mappers
section that lists one or more API
resources. For each resource, the following attributes are specified:
- The
schemaDefinition
that defines the structure of the resource- This references a schema declared in a schema.json file.
- The data model entity that serves as the
root
for mapping information for this resource. - A list of
properties
- Each property includes a
path
attribute. This is a Gosu expression that is used to populate the value of the property. Typically, this expression returns a value from the entity defined in theroot
attribute. - Depending on the nature of the property, there may be additional attributes.
- Each property includes a
For example, the following is a portion of the mapper for the base configuration
Activity
resource:
"Activity": {
"schemaDefinition": "Activity",
"root": "entity.Activity",
"properties": {
"closeDate": {
"path": "Activity.CloseDate"
},
"description": {
"path": "Activity.Description"
},
"mandatory": {
"path": "Activity.Mandatory"
},
...
Note the following:
- This resource is defined in the schema who name is
Activity
(This schema is defined in some other schema.json file.) - The root for the resource mapping is
entity.Activity
. - For each instance of the resource:
- The
closeDate
property is set to theActivity
entity'sCloseDate
field. - The
description
property is set to theActivity
entity'sDescription
field. - The
mandatory
property is set to theActivity
entity'sMandatory
field.
- The
Modifications made to the mapping file
The base configuration includes a set of API-specific extension mapping files. The
purpose of these files is to define mapper information for custom resources for a given
API. These files are named using the pattern
<API>_ext-1.0.mapping.json
, where <API> is the internal name
of the API. For example, the common_ext-1.0.mapping.json
file is used
to define mapper information for custom resources in the Common API. You can access
extension mapping files in Studio through the integration -> mappers -> ext ->
<API>.v1 node.
When you generate endpoints for a custom entity, the REST endpoint generator adds the following code to the relevant extension mapping file:
"<resourceName>": {
"schemaDefinition": "<schemaNameForResource>",
"root": "entity.<customEntity>",
"properties": {
// TODO RestEndpointGenerator : Add mapper properties here
"id": {
"path": "<customEntity>.RestId"
}
}
}
CustomEntity_Ext
entity,
the following is added to the extension mapping
file: "CustomEntityExt": {
"schemaDefinition": "CustomEntityExt",
"root": "entity.CustomEntity_Ext",
"properties": {
// TODO RestEndpointGenerator : Add mapper properties here
"id": {
"path": "CustomEntity_Ext.RestId"
}
}
}
Note that the REST endpoint generator provides information for only one property:
id
. The developer is responsible for:
- Deciding which fields from the custom entity must be available for GETs (and POST/PATCH responses)
- Adding mapper information for those fields.
For example, suppose the CustomEntity_Ext
entity included three
fields:
CustomDescription
(a string)IsActive
(a boolean)ExpirationDate
(a datetime)
The developer decides CustomDescription
and IsActive
must be available to GETs and POST/PATCH responses. The developer would need to add the
code shown below in bold.
"CustomEntityExt": {
"schemaDefinition": "CustomEntityExt",
"root": "entity.CustomEntity_Ext",
"properties": {
// TODO RestEndpointGenerator : Add mapper properties here
"id": {
"path": "CustomEntity_Ext.RestId"
},
"customDescription": {
"path": "CustomEntity_Ext.CustomDescription"
},
"isActive": {
"path": "CustomEntity_Ext.IsActive"
}
}
}
The developer also decides ExpirationDate
must not be available to GETs
and POST/PATCH responses. This field was presumably omitted from the schema. Therefore,
it is also omitted from the mapper.
Mapping syntax for property paths
Scalars
path
property to a field from the data model entity. No other
properties are required. For
example: "description": {
"path": "Activity.Description"
},
Compound datatypes
Typekey
, MonetaryAmount
,
CurrencyAmount
, or SpatialPoint
), you must
include both the path
attribute and an additional
mapper
attribute. The mapper
attribute
specifies the mapper that defines how the compound data type is mapped into its
schema. The following table lists the mapper
attribute values for
the common compound datatypes.Compound datatype | mapper value |
Typekey | #/mappers/TypeKeyReference |
MonetaryAmount | #/mappers/MonetaryAmount |
CurrencyAmount | #/mappers/CurrencyAmount |
SpatialPoint | #/mappers/SpatialPoint |
Activity
data model
entity's AssignmentStatus
field looks like this: "assignmentStatus": {
"path": "Activity.AssignmentStatus",
"mapper": "#/mappers/TypeKeyReference"
},