The REST endpoint generator
The REST Endpoint Generator is a tool that insurers can use to create a set of generated endpoints for a custom data model entity. The tool generates a series of files that define the majority of the functionality for the endpoints. However, the insurer must complete some additional configuration.
- This topic provides an overview of the REST Endpoint Generator.
- For more information on how to run the Rest Endpoint Generator, see Running the REST endpoint generator.
- For more information on configuring the resource definition files, see Configuring the resource definition files.
- For more information on configuring the glue and impl classes, see Configuring glue and impl classes for generated endpoints.
- For more information on configuring authorization, see Configuring authorization for generated endpoints.
REST endpoint generator overview
Insurers typically extend the data model of an InsuranceSuite application with new entities specific to their business model. These data model entities are referred to as custom entities.
Insurers may want to expose some of these custom entities to Cloud API so that caller applications can:
- Retrieve data stored in these entities.
- Create new instances of these entities.
- Modify or delete these entities as needed.
To address this need, Cloud API includes a REST endpoint generator. The REST endpoint generator is a tool that generates a set of CRUD endpoints for a custom entity.
Architecture of custom CRUD endpoints
CRUD endpoints is a term that refers to the endpoints that let you GET a collection of a given resource type and that let you GET, POST, PATCH, or DELETE an element of that resource type. The term "CRUD" is an acronym for Create Read Update Delete.
High-level architecture
The following
diagram depicts the high-level architecture of a set of custom CRUD endpoints within
Cloud API. These endpoints are for a custom entity whose name is
CustomEntity_Ext
.
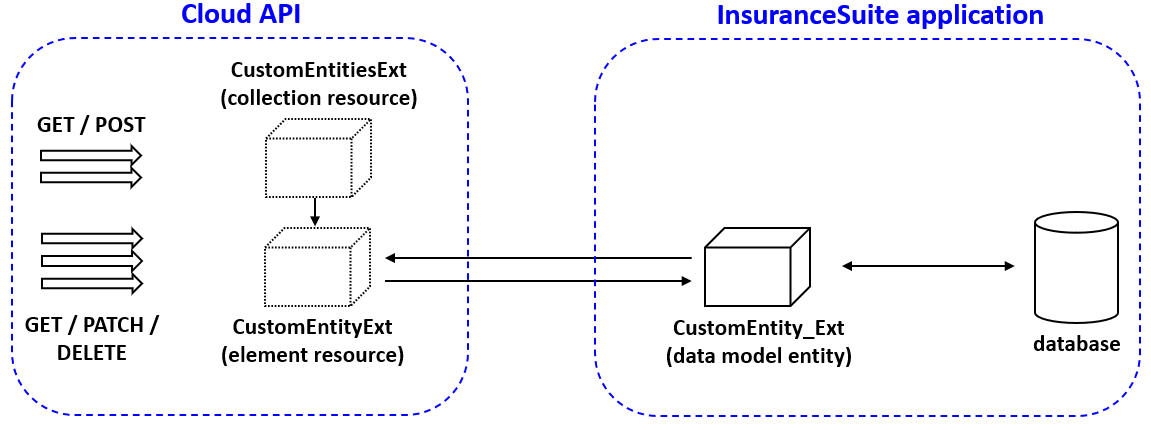
There are five CRUD endpoints, but they do not all interact with the same type of resource.
- The first two CRUD endpoints are for GET (for a collection) and POST. These
endpoints interact with a collection resource whose name is
customEntitiesExt
. (Note that the resource name uses a plural term.) - The final three CRUD endpoints are for GET (for a specific element), PATCH, and
DELETE. These endpoints interact with an element resource whose name is
customEntityExt
. (Note that the resource name uses a singular term.
- The collection resource (
customEntitiesExt
) makes use of the element resource. - The element resource (
customEntityExt
) maps to the data model entity. - The data model entity (
CustomEntity_Ext
) is used to retrieve data from and send data to the database.
Files that define the architecture
The CRUD endpoint architecture is defined in a series of files, as depicted in the following diagram.
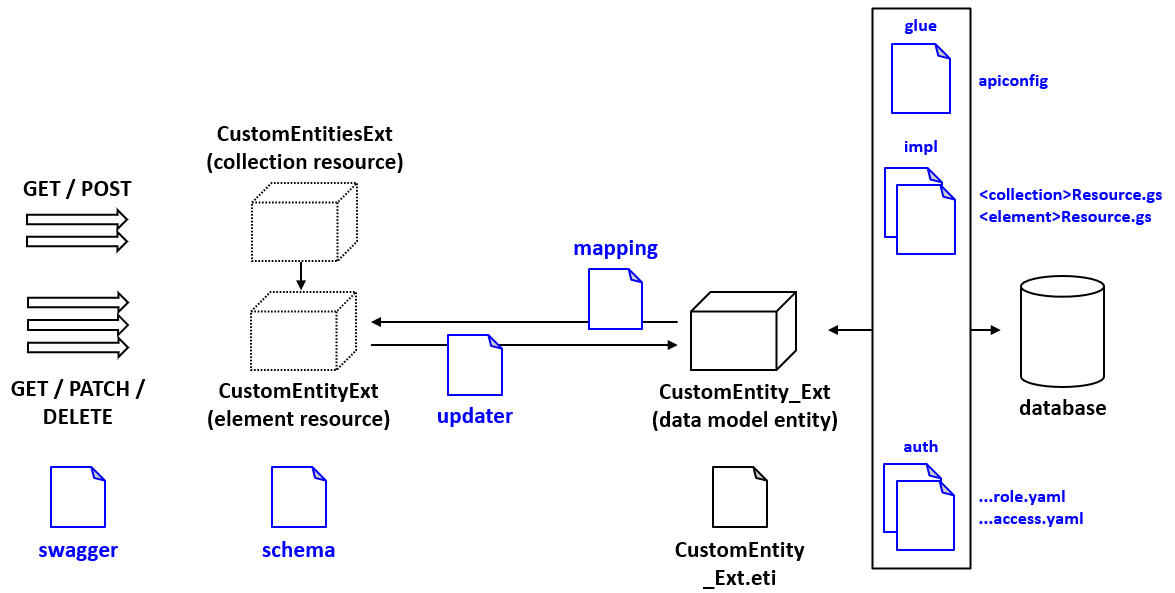
The swagger file defines the endpoints themselves (the paths, operations, and associated resources).
The schema file defines the schema used by the element and collection resources.
The mapping file defines how information is mapped from the data model entity to the element resource. This information is used for GETs, and for the responses of POSTs and PATCHes.
The updater file defines how information is mapped from the element resource to the data model entity. This information is used for POSTs and PATCHes.
The eti file defines the data model entity.
There are also a series of files that define logic for how the endpoints interact with the application.
- The apiconfig file is a "glue" file. One of its purposes is to map both the element resource and collection resource to corresponding "Resource" Gosu files. For collection resources, this file can also specify a default sort order.
- There are two "impl" files that define implementation details.
- The <collection>Resource.gs file is a Gosu file that defines required behaviors for working with collections. This includes behaviors such as how to retrieve the collection from the database.
- The <element>Resource.gs file is a Gosu file that defines required behaviors for working with elements. This includes behaviors such as how to initialize a new element.
- There are two sets of "auth" files that define authorization.
- There are one or more role.yaml files that define endpoint access for callers of the endpoint.
- There are a set of access.yaml files that define resource access for callers of the endpoint.
Architecture and the REST endpoint generator
When you run the REST endpoint generator for a given custom entity, the tool creates
or modifies the files needed to support CRUD endpoints for the custom entity. The
following diagram summarizes the files that are created or modified when running the
tool for an entity whose name is CustomEntity_Ext
.
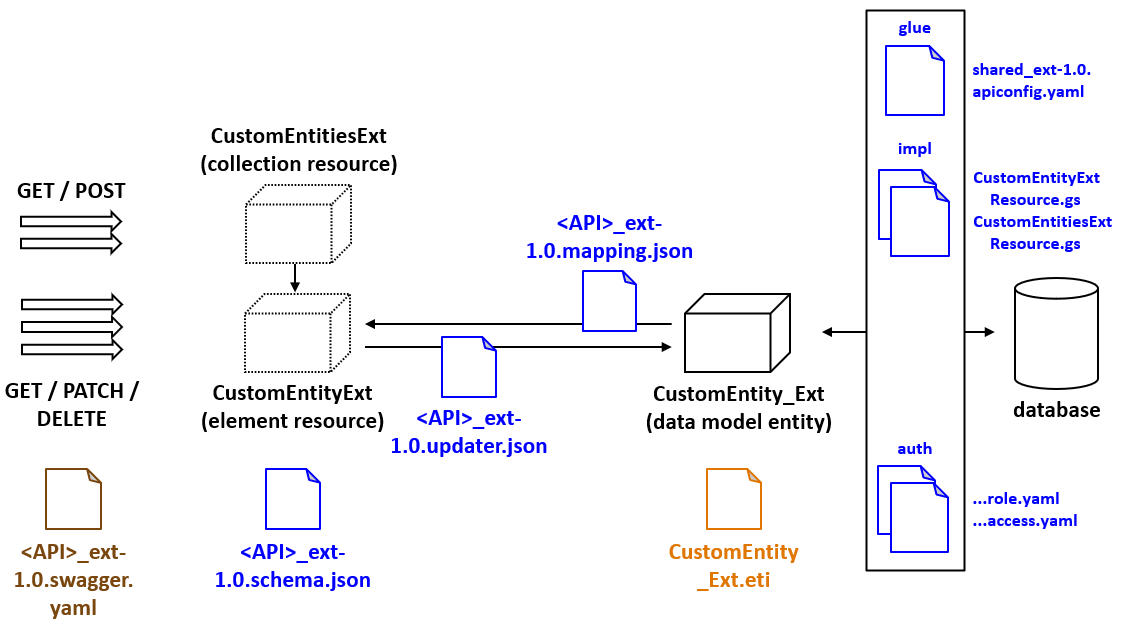
Note the following conventions:
- <API> refers to the name of the API in which the endpoints have been placed.
- The data model entity file (CustomEntity_Ext.eti) appears in orange because it is not created or modified by the REST endpoint generator. It must exist before the generator is run.
- The swagger file (
<API>_ext-1.0.swagger.yaml
) appears in brown because it is modified by the REST endpoint generator, but further configuration is not required. - The remaining files, which appear in blue, are created or modified by the REST endpoint generator. Further configuration to these files is either required or recommended.
REST endpoint generator restrictions
The REST endpoint generator can be used only on non-subtyped custom entities.
- You cannot use the REST endpoint generator for base configuration entities.
- If a base configuration data model entity already has associated endpoints, you can add fields to the corresponding resource. For more information, see Configuring schemas. But you cannot otherwise configure those endpoints.
- However, if a base configuration data model entity does not have any associated endpoints, you cannot use the REST endpoint generator to create endpoints for it.
- You cannot use the REST endpoint generator for custom entities that are subtypes.
When generating custom resources, if the custom entity does not have an "_Ext" suffix, the REST endpoint generator appends an "Ext" or "ext" suffix to the name of the resource in file names and file contents.
The REST endpoint generator generates CRUD endpoints only. It cannot be used to create
business action POSTs, such as an /assign
or /submit
endpoint.
Process for generating CRUD endpoints for a custom entity
About this task
To generate CRUD endpoints for an existing custom entity, you must do the following:
- Run the REST endpoint generator
- Initially, the tool provides a series of prompts. The answers determine how the endpoints are defined.
- Once all prompts have been answered, the tool generates and modifies
files as needed. To help identify where configuration is needed,
the tool adds one or more "
TODO RestEndpointGenerator
" comments to files needing configuration.
- Configure the files that define the resource. This includes:
- Optionally the swagger file
- The schema file
- The mapping file
- The updater file
- Configure the glue and impl Gosu files. This includes:
- The apiconfig.yaml file.
- The element Resource file.
- The collection Resource file.
- Configure the authorization files. This includes:
- The associated role.yaml files.
- The associated access.yaml files.
Special use cases
Integration graphs
An integration graph is a data model graph used by Guidewire App Events. It defines a set of business information to be sent to an external application as part of outbound integrations. For example, the Claim graph defines what information to send about a claim. For more information on integration graphs, see the App Events Guide.
When you generate endpoints for a custom entity, you can also add the custom resource to an integration graph if the resource has a parent resource and that parent resource belongs to the integration graph. For more information, see Additional conisderations for generated endpoints.
Parent entities with additional implications
There is special functionality tied to the Policy entity and all of its child entities. If the Policy entity (or one of its children) is the parent for the custom entity, the REST endpoint generator executes endpoint generation in a special way. For information on these differences, see Additional conisderations for generated endpoints.
Root resources
In most cases, when you generate endpoints for a custom entity, the custom entity is child to some existing parent entity. You access the associated REST resource through that parent entity.
For example, suppose you have a custom entity named
CustomEntity_Ext
. It is a child of the existing
Activity
entity. Information about
CustomEntity_Ext
instances are accessed through the parent
Activity
. In this case, the endpoints have this structure:
- GET
/common/v1/activities/{activityId}/custom-entity-ext
- GET
/common/v1/activities/{activityId}/custom-entity-ext/{CustomEntityExtID}
However, it is possible to generate endpoints for a custom entity as a root resource. In this case, you access the associated REST resource directly.
For example, suppose you have a custom entity named
CustomEntity_Ext
. The endpoints are generated with the associated
resource being a root resource. In this case, the endpoints have this structure:
- GET
/common/v1/custom-entity-ext
- GET
/common/v1/custom-entity-ext/{CustomEntityExtID}
For more information on generating endpoints for root resources, see Additional conisderations for generated endpoints.