Configuring the schema file for generated endpoints
Within the context of Cloud API, a schema file defines the structure of one or more resources. This information is used for GETs, POSTs, and PATCHes.
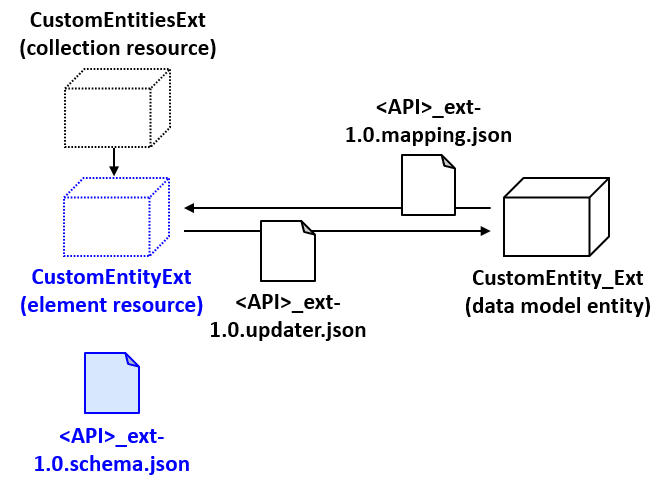
The following sections provide an overview of configuring schema files for generated endpoints. For a complete description of how to configure schema files, see Configuring schemas.
Overview of schema file syntax
A schema file contains a definitions
section that lists one or more
schemas. Each schema is used to structure an API resource. For each schema, the
following attributes are specified:
- A
title
anddescription
, which is used for API definition documentation. - The resource
type
, which is typically set toobject
. - A list of
properties.
Each property includes:- A
title
anddescription
, which is used for API definition documentation. - A
type
, which defines the JSON datatype of the property (for scalar values). - A
ref
, which defines the URI reference for the property (for compound values such as typekeys). - Optional attributes as needed for the business functionality of the property.
- A
For example, the following is a portion of the schema for the base configuration
Activity
resource:
"Activity": {
"title": "Activity",
"description": "An `Activity` is an assignable item that represents a task to be done, a decision to be made, or information to be aware of",
"type": "object",
"properties": {
"activityPattern": {
"title": "Activity pattern",
"description": "The code of the `ActivityPattern` used to create this activity and set its initial values",
"type": "string"
...
}
},
Modifications made to the schema file
The base configuration includes a set of API-specific extension schema files. The purpose
of these files is to define schema information for custom resources for a given API.
These files are named using the pattern <API>_ext-1.0.schema.json
,
where <API> is the internal name of the API. For example, the
common_ext-1.0.schema.json
file is used to define schema
information for custom resources in the Common API. You can access extension schema
files in Studio through the integration -> schemas -> ext -> <API>.v1
node.
When you generate endpoints for a custom entity, the REST endpoint generator adds the following code to the relevant extension schema file:
"<resourceName>": {
"title": "<custom entity name>",
"description": "<custom entity name>",
"type": "object",
"properties": {
// TODO RestEndpointGenerator : Add schema properties here
"id": {
"title": "ID",
"description": "The unique identifier of this element",
"type": "string",
"readOnly": true
}
}
}
For example, if you generate endpoints for a CustomEntity_Ext
entity,
the following is added to the extension schema file:
"CustomEntityExt": {
"title": "Custom entity ext",
"description": "Custom entity ext",
"type": "object",
"properties": {
// TODO RestEndpointGenerator : Add schema properties here
"id": {
"title": "ID",
"description": "The unique identifier of this element",
"type": "string",
"readOnly": true
}
}
}
Note that the REST endpoint generator provides properties
information
for only one property: id
. The developer is responsible for:
- Deciding which fields from the custom entity must be available to the endpoints.
- Adding mapper information for those fields.
For example, suppose the CustomEntity_Ext
entity included three
fields:
CustomDescription
(a string)IsActive
(a boolean)ExpirationDate
(a datetime)
The developer decides CustomDescription
and IsActive
must be available to GETs, POSTs, or PATCHes. The developer would need to add the code
shown below in bold.
"CustomEntityExt": {
"title": "Custom entity ext",
"description": "Custom entity ext",
"type": "object",
"properties": {
// TODO RestEndpointGenerator : Add schema properties here
"id": {
"title": "ID",
"description": "The unique identifier of this element",
"type": "string",
"readOnly": true
},
"customDescription": {
"title": "CustomDescription",
"description": "The description for the CustomEntity",
"type": "string"
},
"id": {
"title": "isActive",
"description": "Whether the CustomEntity is active",
"type": "boolean"
}
}
}
The developer also decides ExpirationDate
must not be available to GETs
and POST/PATCH responses. Therefore, this field is omitted from the schema.
Syntax for property types
The datatype of each property is specified using either a type
attribute
or a $ref
attribute.
Scalars
If a data model entity field is a scalar, set the type
attribute to
one of the JSON schema types from the following table.
Data model datatype | Corresponding JSON type | Additional required properties |
bit | boolean |
|
dateonly | string |
An additional "format " property set to
"date " |
datetime | string |
An additional "format " property set to
"date-time " |
decimal | number |
|
integer | integer |
|
longint | integer |
|
longtext | string |
|
mediumtext | string |
|
money | number |
|
percentage | number |
|
shorttext | string |
|
text | string |
|
varchar | string |
For example, the CustomEntityExt
resource could have the following
scalar properties:
"definitions": {
"CustomEntityExt": {
"title": "Custom entity ext",
"description": "Custom entity ext",
"type": "object",
"properties": {
"id": {
"type": "string"
},
"isActive": {
"type": "boolean"
},
"customDescription": {
"type": "string"
},
"expirationDate": {
"type": "string",
"format": "date-time"
}
}
}
}
Compound datatypes
InsuranceSuite includes several datatypes where multiple values are stored as a unit. This includes the following:
- Typekey (a code and a name)
- MonetaryAmount and CurrencyAmount (a currency and an amount)
- SpatialPoint (a longitude coordinate and a latitude coordinate)
For example, the assignmentStatus
property's datatype is a typekey.
Thus, the response payload for an activity's assignment status has two
sub-fields:
"assignmentStatus": {
"code": "pendingassignment",
"name": "Pending Assignment"
},
When you define a schema property for a compound datatype, do not include a
type
property. In its place, specify a $ref
attribute that specifies an existing definition for the datatype. The following
table lists the $ref
values for the common compound datatypes.
Compound datatype | $ref value | Additional required properties |
Typekey | #/definitions/TypeKeyReference |
A x-gw-extensions attribute with a
typelists child attribute that identifies the
relevant typelist. |
MonetaryAmount | #/definitions/MonetaryAmount |
|
CurrencyAmount | #/definitions/CurrencyAmount |
|
SpatialPoint | #/definitions/SpatialPoint |
For example, the mapping for the Activity
data model entity's
AssignmentStatus
field looks like this:
"assignmentStatus": {
...
"$ref": "#/definitions/TypeKeyReference",
"x-gw-extensions": {
"typelist": "AssignmentStatus"
}
The mapping for the Contact
data model entity's
SpatialPoint
field (which is a spatial point) looks like
this:
"spatialPoint": {
...
"$ref": "#/definitions/SpatialPoint"
}
Additional properties
In a schema file, you must specify the datatype of each property using either a
type
attribute or a $ref
attribute. You can also
add optional attributes. Some attributes are direct children of the property. Others
must be declared inside an x-gw-extensions
object.
Some attributes have default values. The defaults are listed in the following tables.
Additional properties that are direct children of the property
Attribute | Description | Example |
readOnly |
Boolean identifying if the field as read-only. (The default is
false .) |
"readOnly": true |
x-gw-nullable |
Boolean identifying if the field can be explicitly set to null.
(The default is true .) See the following "Marking a
field as required" section for more information. |
"x-gw-nullable": false |
x-gw-sinceVersion |
String identifying the first version of the API to include the property |
"x-gw-sinceVersion": "1.1.0" |
CustomEntity_Ext
entity has an
ExpirationDate
field. The corresponding resource property is
read-only and was added in version 1.1.0. The property declaration would be:
"definitions": {
"CustomEntityExt": {
...
"properties": {
...
},
"expirationDate": {
"type": "string",
"format": "date-time",
"readOnly": true,
"x-gw-sinceVersion": "1.1.0"
}
...
Additional properties declared in the x-gw-extension object
Attribute | Description | Example |
createOnly |
Boolean identifying if the field can be specified only when the
object is created. (The default is false .) |
"createOnly": true |
filterable |
Boolean identifying if the filter query
parameter can be used on this field. In other words, collections can
be filtered using this property. (The default is
false .) |
"filterable": true |
requiredForCreate |
Boolean identifying if the field must be specified when the
object is created. (The default is false .) See the
following "Marking a field as required" section for more
information. |
"requiredForCreate": true |
sortable |
Boolean identifying if the sort query parameter
can be used on this field. In other words, collections can be sorted
using this property. (The default is
false .) |
"sortable": true |
For example, suppose the CustomEntity_Ext
entity has an
SeverityType
field, which is a typekey set to a value in the
SeverityType
typelist. The corresponding resource property must
be specified at create time, cannot be modified after creation, and is both
filterable and sortable. The property declaration would be:
"definitions": {
"CustomEntityExt": {
...
"properties": {
...
},
"severityType": {
"$ref": "#/definitions/TypeKeyReference",
"x-gw-extensions": {
"typelist": "SeverityType",
"createOnly": true,
"requiredForCreate": true,
"filterable": true,
"sortable": true
}
}
...
Marking a field as required by the database
In the data model, some entity fields are required. You cannot create an instance of
the entity without specifying a value for the field. For example, suppose that an
insurer has a business rule stating every activity must have a completion date. To
enforce this, the Activity
entity's EndDate
field
is required.
In a schema, you can mark a resource field as required. To do this, you must set the following properties:
"requiredForCreate": true
- This
x-gw-extensions
attribute indicates the field must be included in POST payloads.
- This
"x-gw-nullable": false
- This attribute indicates that when the field is specified, its value cannot be set to null
The "requiredForCreate": true
expression, by itself, only mandates
that the field must be specified in a POST. There is nothing to prevent a caller
from including the field but setting the field's value to null.
The "x-gw-nullable": false
expression, by itself, only mandates that
if the field is specified, the field's value cannot be set to null.
By combining the two expressions, you are stating that the field must be specified in a POST and set to a non-null value, and anytime thereafter that the field is specified, it must be set to a non-null value. This is the equivalence of setting a data entity field to required.
CustomEntity_Ext
entity has an
CustomDescription
field, which is a string. The field is
required. The property declaration would
be: "definitions": {
"CustomEntityExt": {
...
"properties": {
...
},
"customDescription": {
"type": "string",
"x-gw-nullable": false,
"x-gw-extensions": {
"requiredForCreate": true
}
}
...