Examples
Check out the Usage section for details about how and when to use the Link
component.
Basic link
This example shows the difference between the to
prop and the href
prop. You use to
to specify a target URL within the application, and href
to navigate to both internal and external URLs. When you add the disabled
property, the links are not clickable.
Using the icon
property you can set a custom icon for the link.
import React from 'react';
import { Link } from '@jutro/router/';
export const BasicLink = () => {
return (
<div>
This is an <Link to="/link#examples" icon="gw-bathtub">internal link</Link>.
This is an <Link target="_blank" href="https://docs.guidewire.com/">external link</Link>.
This is a <Link disabled>disabled link</Link>.
</div>
);
};
Managing link events
The Link
component can handle events using the onClick
property when the user clicks on the link, or using onKeyPress
when the user presses a key when the focus is on the link.
import React, { useState } from 'react';
import { Link } from '@jutro/router/';
export const EventsLink = () => {
const [linkText, setLinkText] = useState("Click me!");
const handleClick = () => {
setLinkText("Clicked");
};
const handlePress = () => {
setLinkText("Pressed");
};
return (
<Link
onClick={handleClick}
onKeyPress={handlePress}
>
{linkText}
</Link>
);
};
Link children
You can place any element within a link to have it displayed, as in this example, where a link contains a strong
element. However, adding certain elements such as an input field or a link inside a link might affect accessibility.
import React from 'react';
import { Link } from '@jutro/router/';
export const ChildrenLink = () => {
return (
<div>
<Link>
<strong>This is a child element.</strong>
</Link>
</div>
);
};
Usage
Overview
Link is a component that acts as a navigational element. It can appear in-line with text or on its own. You can attach a link to an icon, image, or text.
When to use
To navigate users to another location, either on the same page or to a different page or site.
When not to use
Do use a link component if the action is purely navigational, including if it takes users to another location where they perform the task.
Don't use a tertiary button for navigation as this could be confusing to users. Tertiary action buttons are more appropriate for performing actions within the current context or page.
Types
There are 2 types of links: standalone and inline.
Standalone links appear on their own or directly after the content.
Inline links appear within a body of text, such as a sentence or paragraph.

Anatomy

The link component consists of the following elements:
- Label: Descriptive text that provides context and explains where the link leads to.
- Icon (optional): Visual indicator that helps users more easily recognize what happens after clicking.
External links
When a link is navigating the user outside of an app, use the gw-open-in-new
icon.
Do use the `gw-open-in-new` icon for external links.
Don't use other icons for external links.
Content
General writing guidelines
- Use sentence case for all aspects of designing Guidewire product interfaces. Don't use title case.
- Use present tense verbs and active voice in most situations.
- Use common contractions to lend your copy a more natural and informal tone.
- Use plain language. Avoid unnecessary jargon and complex language.
- Keep words and sentences short.
Standalone links
Link text should be short and to the point. Use noun phrases, verb phrases, or short descriptive phrases that incorporate relevant keywords related to the link's destination.
Don't use full sentences for standalone links.
Do provide specific link text that helps users know what to expect.
Don't use generic phrases like 'Learn more' or 'Click here' because they lack context and can be ambiguous.
Inline links
Link text must include enough context to communicate the purpose or destination of the link without relying on surrounding context. Many users don't read the adjacent content. For this reason, the terms used in links should be understandable when taken out of context and read alone. Aim for short but meaningful link phrases that indicate the linked content using relevant keywords.
Do use descriptive link text that informs users about the content they'll find.
Don't use vague or generic terms like 'this'.
Try to strike a balance. Link text should be concise yet descriptive enough to make sense out of context.
Don't wrap an entire sentence or text block in a link. This can frustrate screen reader users who have to listen to the entire link.
Do place links at the end of a sentence. This helps to preserve the syntax.
Avoid putting links in the middle of sentences because they can be distracting. They interrupt the flow for people who scan the text, making them stop to read around the link. This can also make reading more challenging for autistic users.
Use meaningful labels
Links must be specific, descriptive, and able to stand alone. Write link text so that even if your users read only the link label, and none of the surrounding text, they can still fully understand what they'll find at that link.
Do use brief but descriptive link text that front-loads the most relevant keywords about the link's destination.
Don't use the URL or domain name as the link label. Both lack clear descriptive value, making it difficult for users to predict where the link will take them.
Be succinct
While there isn't a maximum word count for links, each word included in the link should support the goal of helping users to find what they need. Get to the point so that users will quickly understand the link as they scan and process the page. Try to front-load links with the most important words.
Do be concise and front-load links with information-carrying language.
Don't waste words or defer the information-carrying text to the end.
Link punctuation
Standalone links are not full sentences and don't have punctuation at the end.
If an inline link comes at the end of a sentence, don't include the punctuation mark in the link.
Do treat standalone links as short phrases.
Don't add terminal punctuation to standalone links.
Capitalization
Use sentence case when writing link text. Capitalize only the following words:
- The first letter of the first word in a sentence or phrase
- Proper nouns, such as the names of brands, products, and services
For more information on how to implement sentence case capitalization, see Capitalization.
Do use sentence case for link text.
Don't use title case for link text.
Behaviors
States
The link component has states for enabled, hover, active, focus, disabled, and visited.
Visual | State | Description |
---|
 | Enabled (default) | Communicates to the user that the element is enabled for interaction. |
 | Hover | Provides feedback indicating that the user has placed a cursor over the element (desktop only). Links are underlined when hovered. |
 | Active | Provides feedback indicating that the user is clicking or tapping the element. |
 | Focus | Provides feedback indicating that the user has highlighted the element, typically using an input method such as a keyboard or voice. |
 | Disabled | Communicates to the user that the element is not currently available for interaction. |
 | Visited | Indicates that the user has previously clicked on and viewed the linked content. |
Interactions
Mouse
Users can open the link by clicking anywhere on the label or the associated icon.
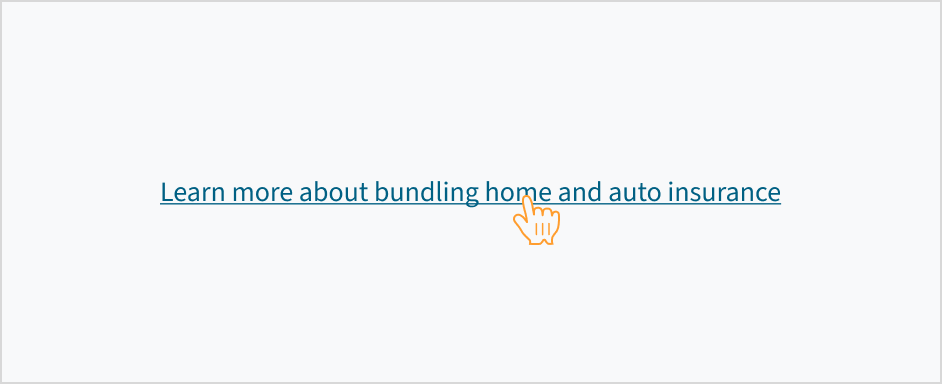
Keyboard
Pressing the Enter
key on a focused link activates the link and moves focus to the link destination.
Screenreader
On focus, the role 'link' is voiced by a screen reader, as is its textual value (for example, 'Link, more information'). When you use the disabled link, the ARIA state is set to aria-disabled='true'
. The state is voiced by a screen reader as 'dimmed/not available'. When the link becomes active, the aria-disabled
state changes to 'false'.
Accessibility
The Jutro link component is a native HTML hyperlink element.
This component adheres to the following criteria for color and zoom accessibility:
- Color: The contrast ratio of textual elements against their background is above 4.5:1 as per WCAG 2.0 AA requirements.
- Zoom: All content is visible and functional up to and including a zoom factor of 200%. On focus, the link text becomes underlined and is surrounded by a border. This helps users understand the current focus position.
This component has been validated to meet the WCAG 2.0 AA accessibility guidelines. However, changes made by the content author can affect accessibility conformance. Follow the guidance below when using this component in your applications:
- Ensure that any color changes meet the required threshold of 4.5:1.
- Avoid using words and phrases such as “Click here” or “here” as link text. Instead, create meaningful link text that makes sense on its own.