Dropdown
Usage
Overview
The dropdown component presents users with a set of options from which they select. Dropdowns progressively disclose options, preventing users from seeing too much information at one time. They can also be used to group information. Dropdowns are always supported by a field label or title that is positioned before the control.
This component comes in four variants: select, multiple select, combobox, and multiple combobox.
When to use
- In forms on full pages, in modals, or on side panels.
- To filter or sort contents on a page.
- When one option can be selected, use select or combobox.
- When more than one option can be selected at the same time, use multiple select or multiple combobox.
When not to use
There's a small number of options (5 or fewer) from which users can choose. Use the radio group for small sets of mutually exclusive items or checkboxes for multiselect items.
Source: Nielsen Norman Group
Formatting
Anatomy
Example of the dropdown component, select variant.
- Label: Describes the purpose of the input field.
- Help text (optional): Gives extra context or helps the user choose the right selection.
- Dropdown input: Displays the user selected option or placeholder text.
- Dropdown menu: Displays a list of options to choose from.
Typeahead
Jutro accommodates dropdown menus with typeahead input fields. Users can manually enter text to filter the dropdown items. This feature is useful for helping users select from a long list.
Dropdowns with typeahead enabled provide suggestions as users begin typing. Selections appear based on the characters that users have entered. The more characters that users input into the field, the more refined the list becomes.
For dropdown variants that support searching and filtering, see the combobox and multiple combobox.
Order of options
When using the dropdown for sorting purposes, consider arranging options in order. For example:
- From most common to least common option
- From simplest to most complex operation
- From least to most risky option
The first option would appear at the top of the list.
Avoid arranging the list of options alphabetically unless it makes sense for your use case. Lists of countries and other known-item problems are often fine to alphabetize. However, you do need to ensure that users will know unambiguously the name of their selection.
Source: Nielsen Norman Group
Content
General writing guidelines
- Use sentence case for all aspects of designing Guidewire product interfaces. Don't use title case.
- Use present tense verbs and active voice in most situations.
- Use common contractions to lend your copy a more natural and informal tone.
- Use plain language. Avoid unnecessary jargon and complex language.
- Keep words and sentences short.
Include a label
Place the dropdown label outside of the field so that it's always visible. A dropdown without a label is not accessible.
Don't replace field labels with in-field placeholder text. This hurts usability and has many negative consequences.


Keep menu items concise
Limit each dropdown option to one line of text. If the text is too long for one line, you can add an ellipsis (...) for overflow content, and accompany with a browser-based tooltip to show the full string. However, it's recommended that you avoid truncation if at all possible.
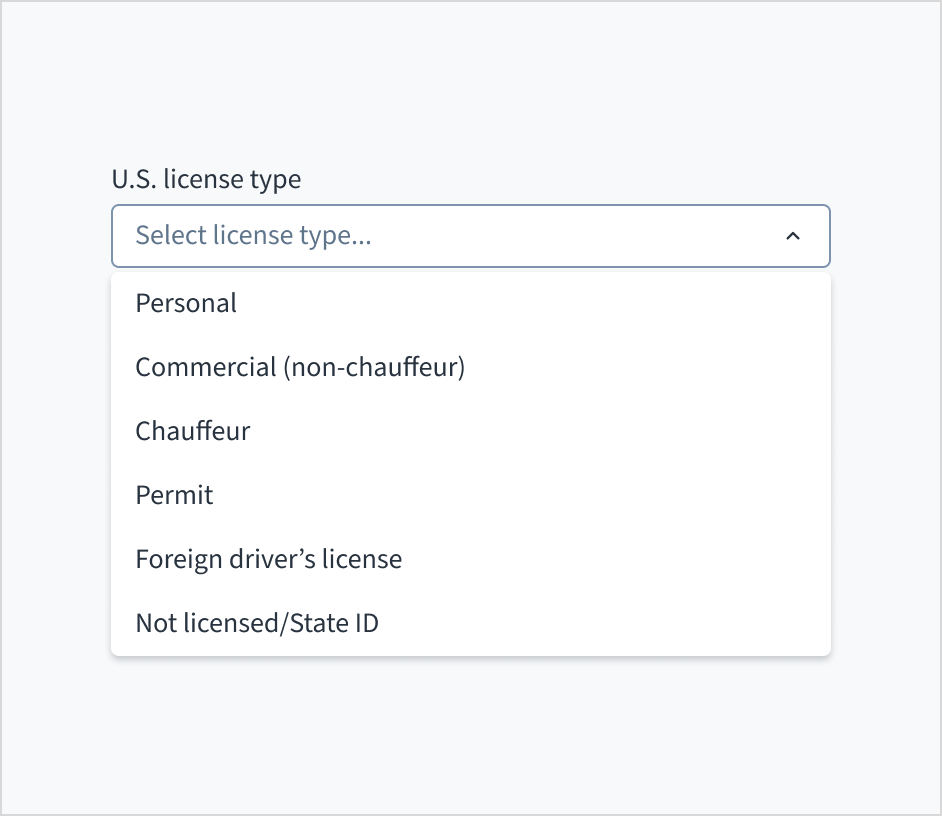

Cut filler words
Cut filler words like “please” to reduce word and character counts and make content easier to absorb.
“Please” is often overused, so we reserve it for specific situations. For example:
- There's a problem that we can't help the user solve.
- The user is asked to do something inconvenient.
- The software is to blame for the situation.
If you're simply prompting a user to select an option from the dropdown menu, you wouldn't preface that with “please.”
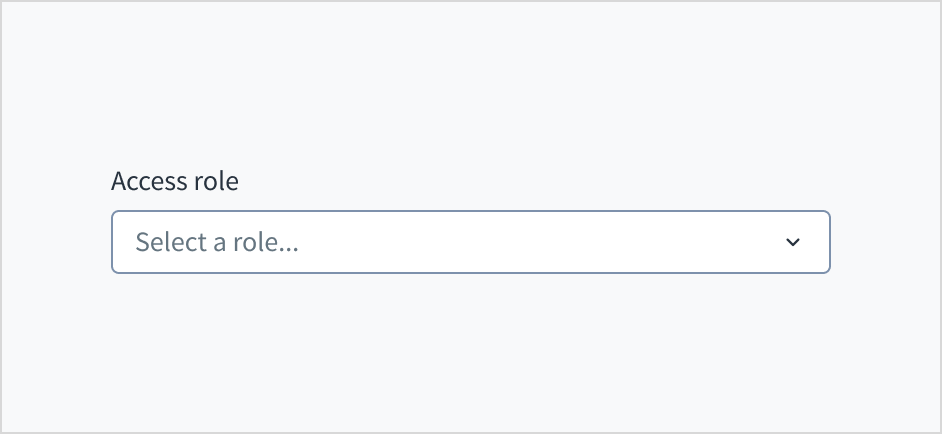
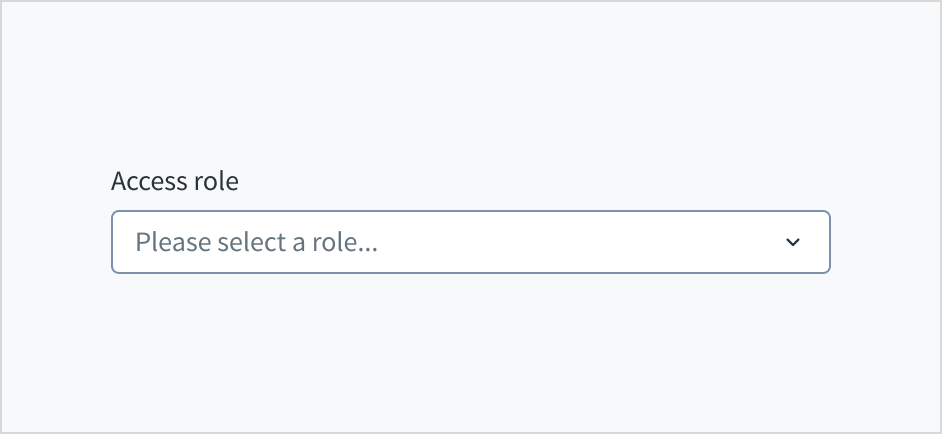
Add help text if it's meaningful
Use help text to show context and communicate what to select or how to select an option. Here are some examples of what you might include in help text:
- An overall description of the dropdown options
- Hints that assist the user in choosing the right selection
- More context for why a user needs to make a selection
Only use help text for pertinent information. Avoid using help text that simply restates the same information that appears in the label.
Use sentence case for help text. Write 1-2 short, complete sentences that end with a period.
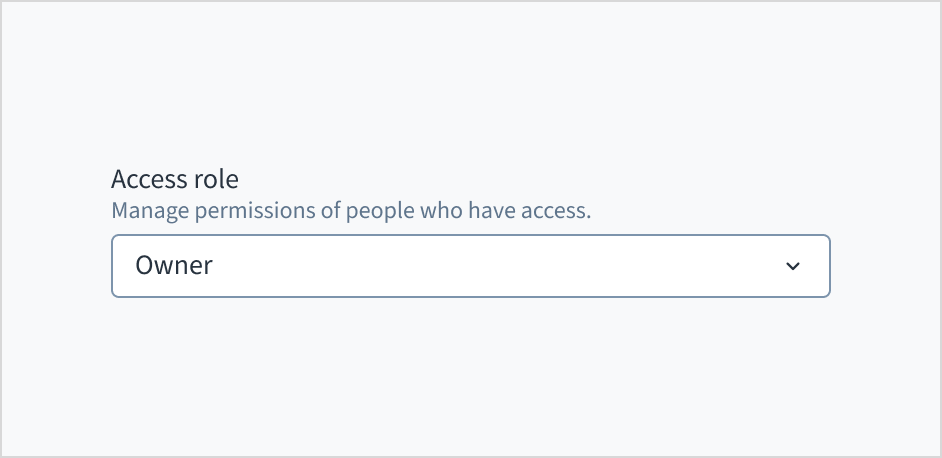
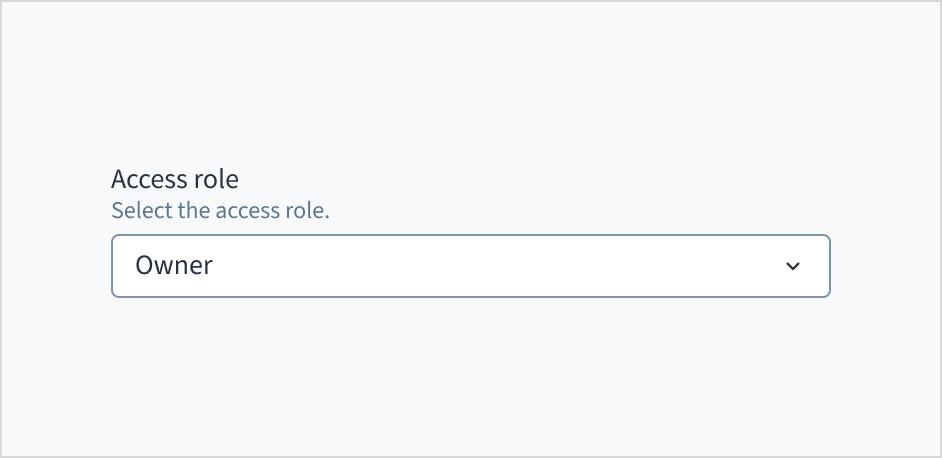
Use error text to guide users
Error message text tells a user how to fix the error. In the case of the dropdown, errors are often related to something that must be fixed for in-line validation. For example, if someone doesn't select an employment status and this is a required field, you can use error text to guide the user to a solution: “Select an employment status.”
Use sentence case for error text. Write 1-2 short, complete sentences that end with a period.


Mark required fields
Use an asterisk (*) to indicate required fields. The asterisk precedes the field label. This helps users to easily locate which fields are required by scanning just the left-most character of the label.

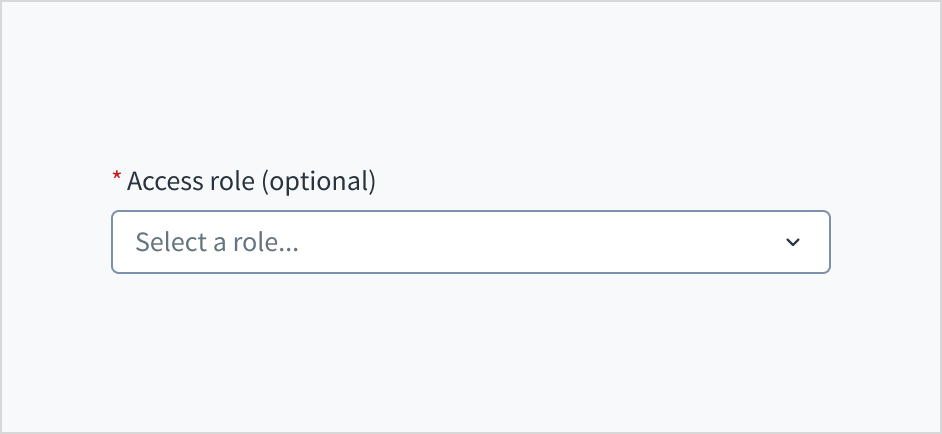
Use sentence case
Field labels, placeholder text, and menu items appear in sentence case.
Refer to the UI text style guide for more information on how to implement sentence case.
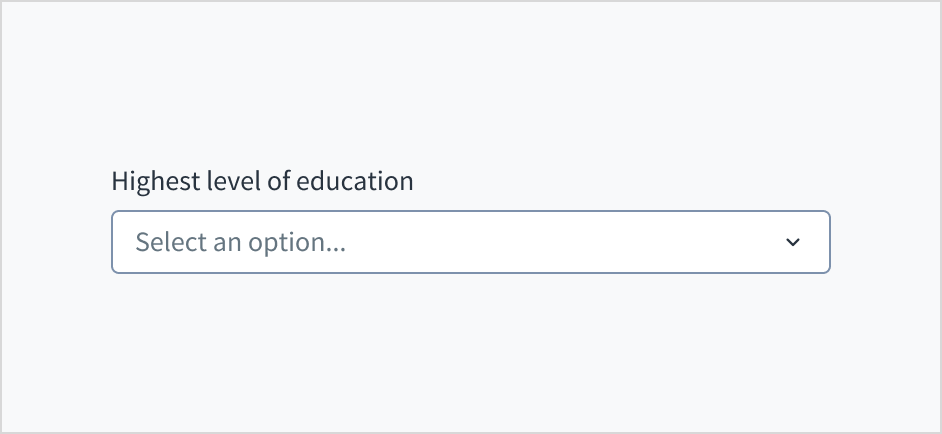
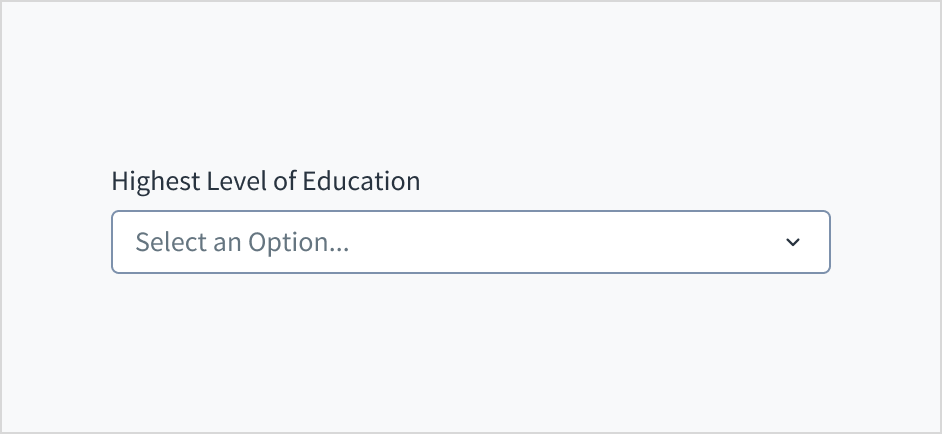
Behaviors
All dropdown variants share the behaviors listed in this section. For behaviors that are unique, refer to the usage guidelines for each variant.
States
The dropdown field appears with no value (default), placeholder text, or a filled input.
Visual | State | Description |
---|---|---|
No value (default) | Indicates to the user that no value has been selected and there is no placeholder. | |
Placeholder | Indicates to the user that no value has been selected. The placeholder is grayed out. | |
Filled input | Indicates to the user that the input is filled with data. |
Dropdowns also have interactive states for enabled, focus, disabled, error, read-only, and display-only.
State | Description |
---|---|
Enabled | Indicates to the user that the element is enabled for interaction. |
Focus | Indicates to the user which UI element in the system is focused. |
Disabled | Indicates to the user that the input value can't be changed because of local factors. For example, a checkbox above the input field must be checked to access this input field. The user can take action to enable it by interacting with the page. |
Error | Indicates that the user has made a validation error. Error text provides corrective feedback to users. |
Read-only | Indicates to the user that the input value can't be changed because of outside factors. For example, lack of write access. The user can take action to enable it by, for example, contacting an administrator. |
Display-only | The display-only state is used for two cases:
|
The following image illustrates dropdown interactive states.
Interactions
Mouse
Users can interact with this element by clicking in the area inside border.
Keyboard
The dropdown is expanded and collapsed using the Spacebar. Users navigate through the options using the arrow keys and select by means of the Spacebar or Enter key.
Screenreader
The aria-labelledby
establishes a programmatic association between the input field and its label. The WAI-ARIA attribute of aria-autocomplete='list'
communicates that a list of choices will appear from which the user can choose, but the edit box retains focus. Selecting the 'required' option in Storybook adds both the 'required' and 'aria-required="true"' attributes to the input field.
Accessibility
This component adheres to the following criteria for color and zoom accessibility:
- Color: The contrast ratio of textual elements against their background is above 4.5:1 as per WCAG 2.0 AA requirements.
- Zoom: All content is visible and functional up to and including a zoom factor of 200%.
This component has been validated to meet the WCAG 2.0 AA accessibility guidelines. However, changes made by the content author can affect accessibility conformance. When using this component within your application:
- Assist users in understanding the content by avoiding very long option names.
- Avoid using implicitly focusable elements such as buttons, checkboxes and links, or implicitly semantic content such as headings within your dropdown components.