Button
There are deprecated versions of this component. Switch to a version of the docs older than 10.0.x to view their docs.
Examples
Check out the Usage section for details about how to design a button properly, and the different alternatives and variants provided. Using the available properties you can create different variants of a button.
Simple button
The Button
component is made available from the @jutro/components
package. There are 4 basic properties when working with the button: label, icon, variant and onClick. In most cases, these will be the only properties you will use.
import Button from '@jutro/components';
// ...
<Button
label="Click me"
icon="gw-bathtub"
onClick={handleCLick}
/>;
Icon only button
Using the hideLabel
property you can create an Icon only button that does not display a label, but only the chosen icon. The label
property will be used for the aria-label
of the button.
<Button
icon="gw-bathtub"
label="this will be the aria-label text"
onClick={handleClick}
hideLabel
/>
In this case, the iconPosition
property value will be ignored and the icon will be always displayed centered.
Async button
When a button triggers an action and it needs to wait for its completion or to a specific event happening, it is possible to handle it:
import React, { useEffect, useState } from 'react';
import { InlineLoader } from '@jutro/components';
import { Button } from '@jutro/components';
import styles from './AsyncButton.module.css';
function getFromAPI() {
return new Promise((resolve) => {
setTimeout(() => {
resolve('Done!');
}, 2000);
});
}
export function AsyncButtonExample() {
const [loading, setLoading] = useState(true);
useEffect(() => {
async function someAsyncFunction() {
await getFromAPI();
setLoading(false);
}
if (loading) {
someAsyncFunction();
}
}, [loading]);
return (
<Button
label={loading ? 'Saving' : 'Save'}
renderIcon={() => <InlineLoader loading={loading} />}
iconPosition="right"
disabled={loading}
onClick={() => setLoading(true)}
/>
);
}
Button with tooltip
Tooltip
is added through composition: Tooltip
+ Button
<Tooltip content="This is a tooltip">
<Button label="Click me" />
</Tooltip>
There are deprecated versions of this component. Switch to a version of the docs older than 10.0.x to view their docs.
Usage
Overview
The button is a component that enable users to perform actions and make choices, with a single click. Think of the button as facilitating a conversation between the user and the experience. Most of the time when users encounter text—for example, in an empty state, confirmation, or error—the experience is speaking to the user. Buttons are among the most important components that users interact with. They let users make their purpose known.
When to use
- To trigger an action. For example, Submit, Upload, Add, Delete, Save, or Apply.
When not to use
- For navigating to new page. Use the link component instead.
- For uploading files. Use the file uploader component instead.
Formatting
Anatomy
Buttons consist of the following elements:
- Primary buttons display containers (A) around a text label (B) with an optional icon (C). Primary buttons have a solid background color.
- Secondary buttons display containers (A) around a text label (B) with an optional icon (C). Secondary buttons have a visible stroke and no background color.
- Tertiary buttons display a text label (B) with an optional icon (C).
- Icon buttons display containers (A) around a mandatory icon (C).
Alignment and placement
When a button group appears as part of a process, such as in a wizard or in a modal, we recommend placing them in the bottom right corner of the page to align with the natural flow of pages. Inside that button group, primary buttons go on the far right.
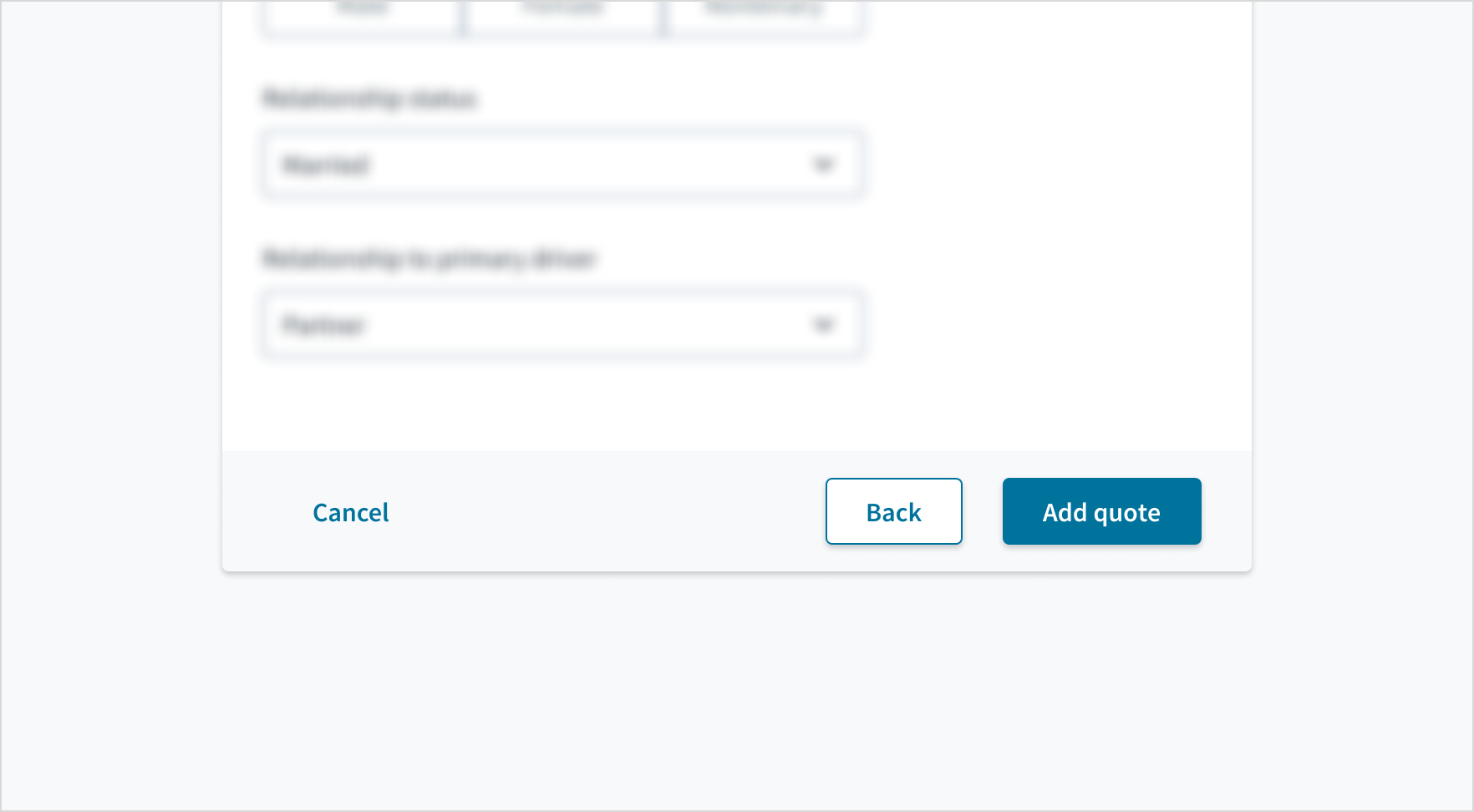
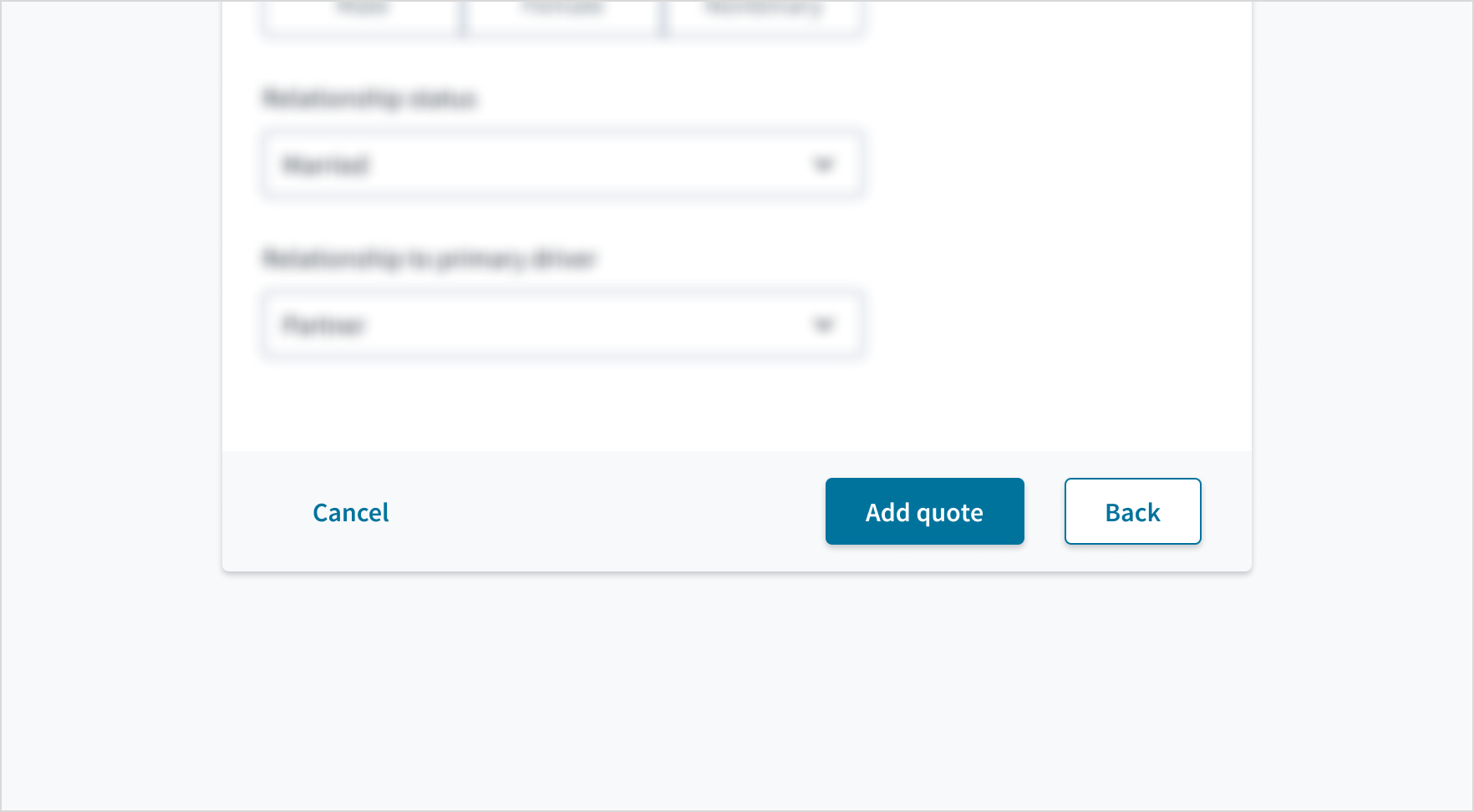
For customer configuration, you can set the icon to the left of the button label or the right, but not both at the same time.
For internal Guidewire applications, always position the icon on the right so that the user reads the label first, then sees the icon.

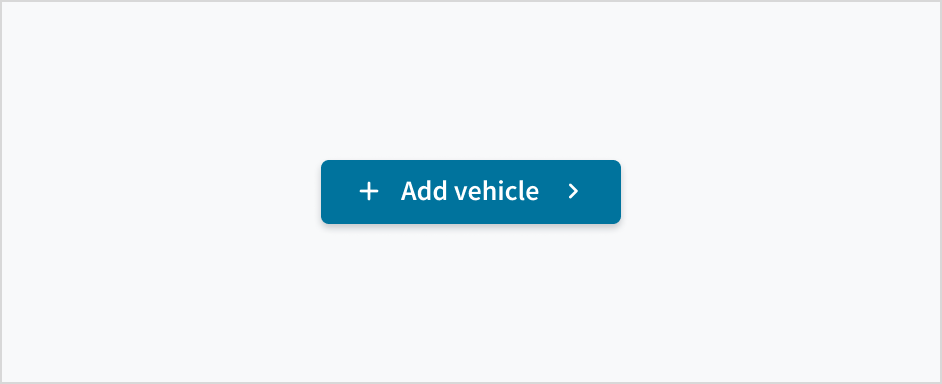
Button types
Buttons are rectangular with radiused corners, drop shadows (except for tertiary), simple color treatments, and text labels that use sentence case.
Type | Purpose |
---|---|
Primary button | Primary buttons are high emphasis. Use them for the most important action. Each page must have only one primary button to trigger the action (not including modal or quick view). |
Secondary button | Secondary buttons are medium emphasis. Use secondary buttons in conjunction with a primary button. As part of a pair, the secondary button's function is to perform the dismissive action of the set. They offer a way for the user to back out and take no action. For example, Cancel versus the primary action Send. Don't use a secondary button in isolation and don't use a secondary button for the main action. |
Tertiary button | Tertiary buttons are low emphasis. Use them for less pronounced, and sometimes independent, actions. You can use tertiary buttons in isolation or pair them with a primary button when there are multiple calls to action. When a primary button for the main action is present, you can also use tertiary buttons for sub-tasks on a page. |
Icon button | Icon buttons are intended for widely recognized actions and must appear in conjunction with a tooltip. |
Each page must have only one primary trigger action:
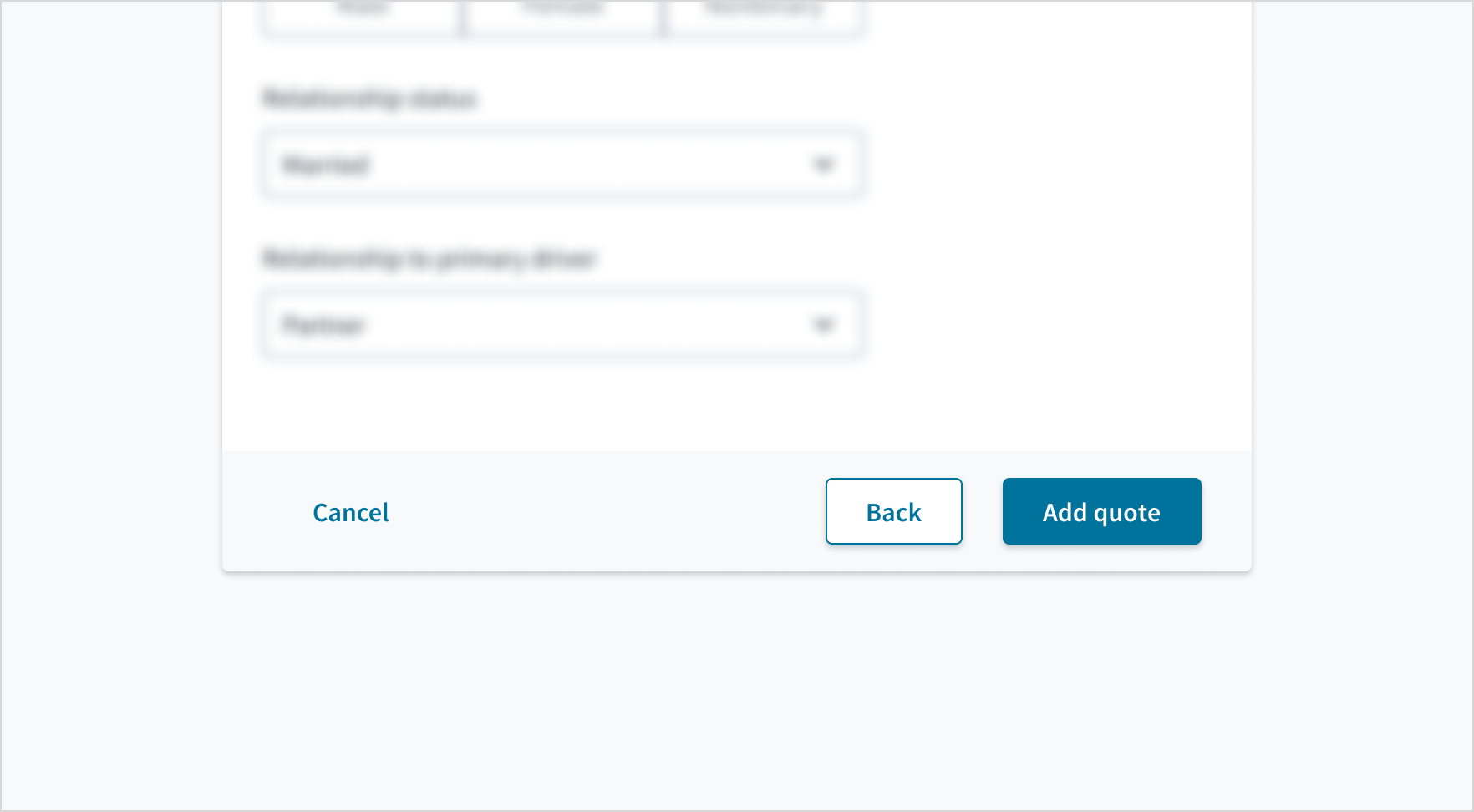
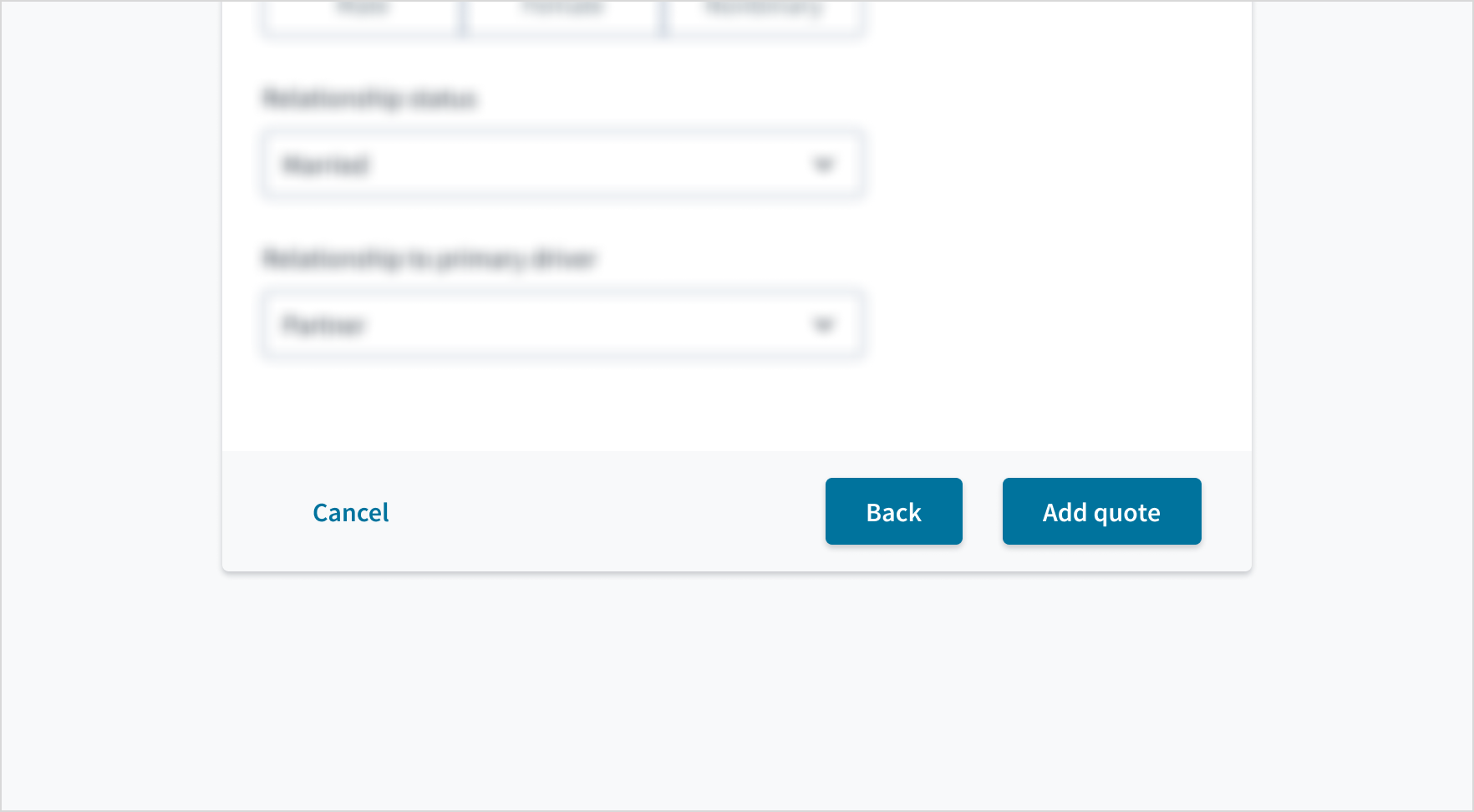
Button groups
Buttons can be grouped to show the relationship between them. We recommend using a 16px gap between buttons (both vertically and horizontally).


Content
General writing guidelines
- Use sentence case for all aspects of designing Guidewire product interfaces. Don't use title case.
- Use present tense verbs and active voice in most situations.
- Use common contractions to lend your copy a more natural and informal tone.
- Use plain language. Avoid unnecessary jargon and complex language.
- Keep words and sentences short.
Be concise
- Keep button labels short and simple: 1 or 2 words, no longer than 4 words.
- Remove articles (“a”, “an”, and “the”) to support scannability, comprehension, and task completion.
Reference: Nielsen Norman
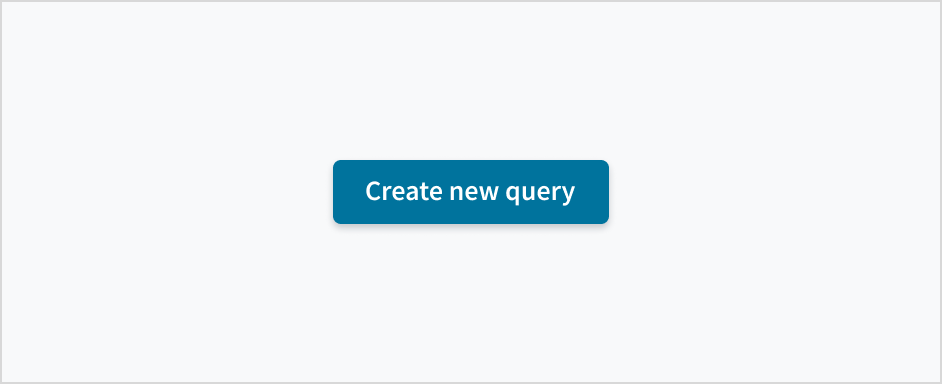
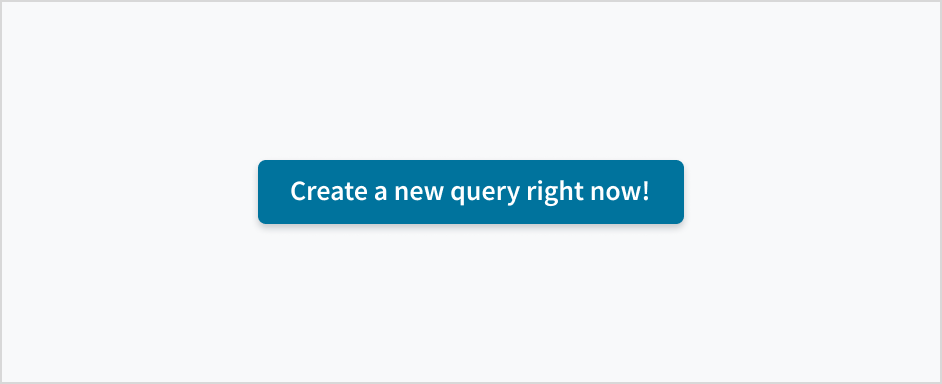
Clearly state the action
- For commands that initiate an action or submit information, start button labels with a verb. If the resulting action is not clear from the verb alone, use a noun after the verb to disambiguate the context. For example, Add vehicle instead of Add, or Create schedule instead of Create.
- Don't use Yes and No for button labels. Name the action that happens when the user clicks.
- Choose words that logically align with the preceding content: If your headline asks Delete ## object type? then your button text must also say Delete.
- Take care when using a primary button for a destructive action, especially when it can be triggered by keyboard return. In these instances, be very explicit so there's no ambiguity.
For consistency, see Jutro's content guidelines for a list of recommended action labels.

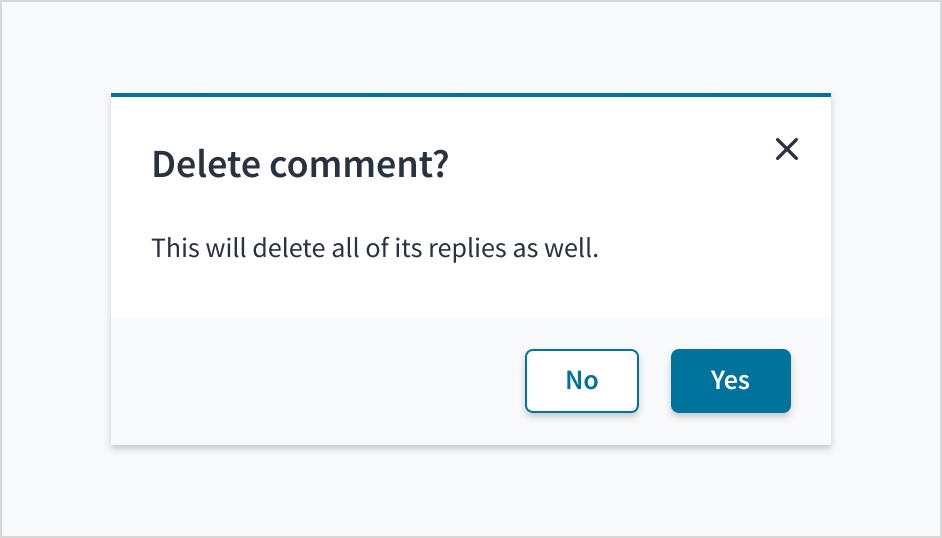
Use sentence case
Button text must always be in sentence case. Never use all caps to emphasize a specific button, and don't write in title case.
Refer to the UI text style guide for more information on how to implement sentence case.

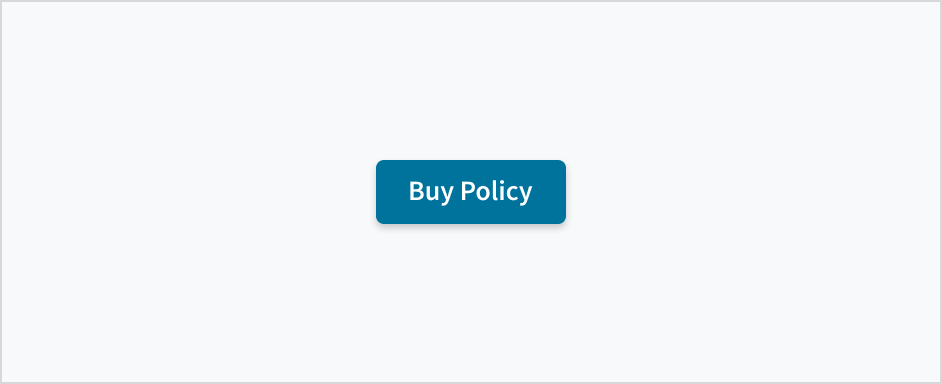
Be mindful of tone
- Button labels should tap into users' existing vocabulary. Ask yourself, “Is this a word that a person would actually say in a conversation?”
- Don't use emojis, exclamation points, or other forms of punctuation in button labels.
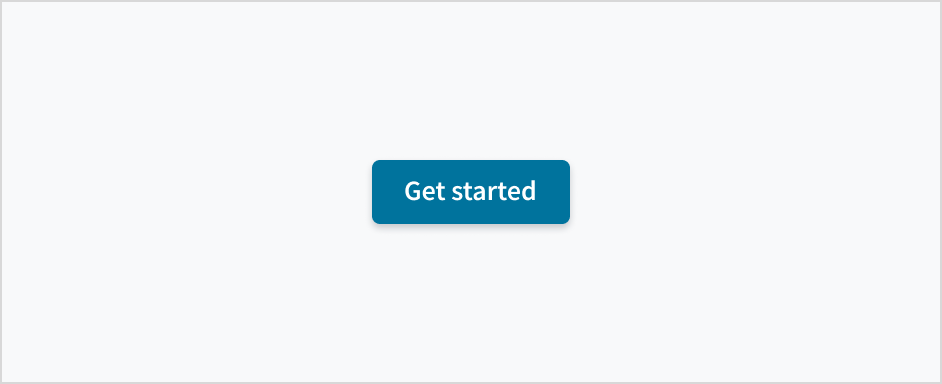
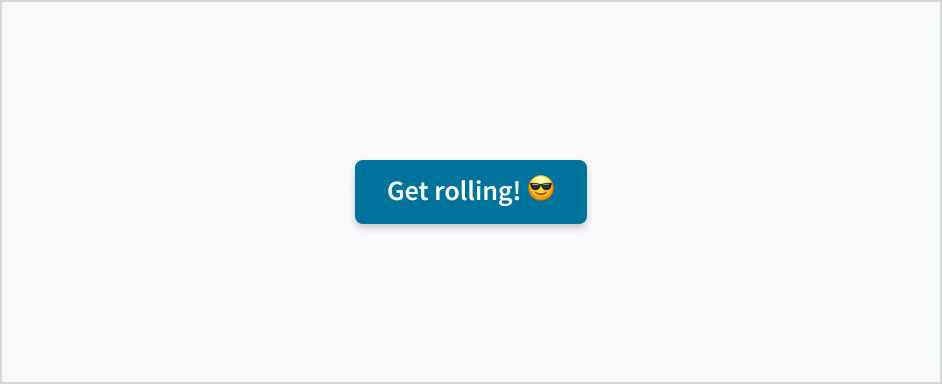
Behaviors
States
Buttons have states for enabled, hover, focus, active, disabled, and loading.
State | Description | |
---|---|---|
Enabled | Communicates to the user that the element is enabled for interaction (default). | |
Hover | Indicates that the user has placed a cursor over the element (desktop only). The Hover state can be used with the Enabled and Loading states. | |
Focus | Indicates that the user has highlighted the element, typically using an input method such as keyboard or voice. The Focus state can be used with all other states (including Disabled). | |
Active | Indicates that the user is clicking or tapping the element. The Active state can be used with the Enabled and Hover states. | |
Disabled | Communicates to the user that the element is not interactive. Every button is Accessible Disabled by default. That means the disabled button can be focused. | |
Loading | Communicates to the user that the action is in progress. Use the Loading state when an action is supposed to take more than 1 second. When a button is in the Loading state, the following must happen:
|
Interactions
Mouse
The button can be triggered by clicking anywhere inside the button container.
Keyboard
When the button has focus, both Space and Enter will activate it.
Screen reader
The button component uses the implicitly semantic HTML5 <button>
tag. Text within the tags acts as the accessible name.
Responsiveness and adaptiveness
Jutro buttons come in 2 different sizes to accommodate the needs of both enterprise and consumer applications. Enterprise applications require greater data density, and as a result, benefit from smaller buttons and more screen real estate. Like most components, buttons can be distributed, sized, and arranged by our Grid
and Flex
components as part of Jutro's commitment to responsive design.
Small button | Medium button |
---|---|
In mobile experiences, we recommend filling the container width with buttons (the text and icon inside of the button must be centered).
Accessibility
The Jutro button element represents an HTML button that is used to submit information. Text between the opening and closing tags appears as the text of the button.
- Color: The contrast ratio of the text against its background is above 4.5:1, as per WCAG 2.0 AA requirements.
- Zoom: The button is responsive. All content is visible and functional at a zoom factor of 200%.
This component has been validated to meet the WCAG 2.0 AA accessibility guidelines. However, changes made by the content author can affect accessibility conformance. Follow the guidance below and refer to the WAI-ARIA Authoring Practices for Buttons when using this component in your applications:
- The button text must clearly describe the action of the button.
- If applying custom color themes, check that the contrast ratio of text against its background meets the required threshold.
Accessibly disabled component
The Button
component remains accessibility compliant, even when disabled, as of Jutro version 8.4.0. This functionality is enabled by default for every new app that is created using version 8.4.0 of Jutro or later. Older apps can employ this functionality by adding the accessibleDisabled
object to their config.json
file and passing props, as shown below.
Accessible disabled Button
components have the following characteristics:
- The
disabled
HTML attribute is removed. - An additional property called
aria-disabled
is defined. - All callback functions, such as
onClick
andonBlur
, are muted and unable to be called. - The HTML type attribute value of
Button
will be changed tobutton
. - If the button has the
href
attribute provided, then its role will be changed tolink
and itstabIndex
will be changed to 0.
Styling for accessible disabled and standard disabled buttons remains the same.
There are deprecated versions of this component. Switch to a version of the docs older than 10.0.x to view their docs.
Code
<Button
label="Click me!"
onClick={handleClick}
/>
Import statement
import { Button } from '@jutro/components';
Component contract
Make sure to understand the Design System components API surface, and the implications and trade-offs. Learn more in our introduction to the component API.
Properties
label
required- Type
IntlMessageShape
DescriptionLabel displayed on the button, also passed as a default value to 'aria-label'
className
- Type
string
DescriptionClass passed to the button element
disabled
- Type
boolean
Default valuefalse
DescriptionIf set to true button is disabled
IMPORTANT: this prop overrides the native HTML disabled attribute. In this case button is still tabbable, even when it's disabled
fullWidth
- Type
boolean
Default valuefalse
DescriptionIf set to true the button will take the full width of its parent container
hideLabel
- Type
boolean
Default valuefalse
DescriptionIf set to true label is not displayed
icon
- Type
string
DescriptionIcon name, for instance
gw-search
iconPosition
- Type
left | right
Default valueleft
DescriptionSets where the icon is placed relative to the text
onClick
- Type
React.MouseEventHandler
DescriptionCallback called when button is clicked
renderIcon
- Type
React.FC
DescriptionRenders custom icon
size
- Type
small | medium
Default valuesmall
DescriptionSets the size of button (small, medium)
variant
- Type
primary | secondary | tertiary | neutral | inverse
DescriptionSets the variant of button to display (primary, secondary, tertiary, neutral, inverse)
Note: neutral and inverse variants are applicable to the button with icon only
default: primary
Hooks
No hooks are available for Button.
Translation keys
There are no translations for Button.
For information on how to manage translations, see our section about Internationalization.
Escape Hatches
For more information, see our documentation about escape hatches.
Passing HTML properties to the component
You can use HTML button attributes, except any of the ones which are overridden by Jutro.
<Button
label="Click me"
onClick={handleClick}
id="my-button"
variant="primary"
/>
The result of passing this id
property will be that it will be part of the HTML button that is created in the HTML DOM.
Button custom behaviors
Accessible disabled component
The Button
component remains accessibility compliant, even when disabled.
Accessible disabled Button
components have the following characteristics:
- The
disabled
HTML attribute is removed. - An additional property called
aria-disabled
is defined. - All callback functions, such as
onClick
andonBlur
, are muted and unable to be called. - The HTML type attribute value of
Button
will be changed tobutton
. - If the button has the
href
attribute provided, then its role will be changed tolink
and itstabIndex
will be changed to 0.
Styling for accessible disabled and standard disabled buttons remains the same.
Legacy information
Are you using one of the legacy buttons?
- To view docs for the old components, switch to a version of the docs older than 10.0.x.
- These legacy components are also available in Storybook:
Changelog
10.0.0
New @jutro/components/Button
component introduced replacing previous one.
Previous button-related components were deprecated and moved to the @jutro/legacy
package. To view their documentation switch to an older version.
Component | Old location | New location |
---|---|---|
Button | @jutro/components/Button | @jutro/legacy/components/Button |
IconButton | @jutro/components/IconButton | @jutro/legacy/components/IconButton |
AsyncButtonLink | @jutro/router/AsyncButtonLink | @jutro/legacy/router/AsyncButton |
ButtonLink | @jutro/router/ButtonLink | @jutro/legacy/router/ButtonLink |
Codemods are available to automatically rename existing imports.