There are deprecated versions of this component. Switch to a version of the docs older than 10.0.x to view their docs.
Examples
Simple Accordion
<Accordion>
<AccordionCard title="Card 1">Test card</AccordionCard>
<AccordionCard
title="Card 2"
initialExpanded>
Card initially open
</AccordionCard>
<AccordionCard
disabled
title="Card 3">
Test card 3
</AccordionCard>
</Accordion>
The Accordion
and AccordionCard
components are available from the @jutro/components
package. Only AccordionCard
can be placed inside Accordion
. AccordionCard
always has to have set either a title
or dangerouslyRenderHeader
prop but the usage of the latter is not recommended.
Accordion appearance customization
<Accordion
chevronPosition="left"
hideDivider>
<AccordionCard title="Card 1">Test card</AccordionCard>
<AccordionCard title="Card 2">Test card 2</AccordionCard>
<AccordionCard
disabled
title="Card 3">
Test card 3
</AccordionCard>
</Accordion>
Using the hideDivider
property allows you to hide all of the separators. Chevron placement is limited to right
and left
options. Both are set on the Accordion
level.
.customHeaderContainer {
display: flex;
align-items: center;
}
.customHeaderIcon {
margin-right: 8px;
}
import {
Accordion,
AccordionCard,
useAccordionCardState,
} from '@jutro/components';
const CustomHeader1 = () => {
const { expanded, title } = useAccordionCardState();
return (
<div className={styles.customHeaderContainer}>
{expanded ? `Expanded ${title}` : `Collapsed ${title}`}
</div>
);
};
const CustomHeader2 = () => {
const { expanded } = useAccordionCardState();
return (
<div className={styles.customHeaderContainer}>
{expanded ? (
<Icon
icon="gw-add"
className={styles.customHeaderIcon}
/>
) : (
<Icon
icon="gw-alarm"
className={styles.customHeaderIcon}
/>
)}
Custom rendered card header
</div>
);
};
<Accordion>
<AccordionCard title="Card 1">Test card</AccordionCard>
<AccordionCard
title="custom card header"
dangerouslySetHeader={<CustomHeader1 />}>
I'm basic but custom
</AccordionCard>
<AccordionCard
dangerouslySetHeader={<CustomHeader2 />}
initialExpanded
onFocus={() =>
console.log(
"I'm a custom attribute of a button added to be triggered on accordion card button focus"
)
}>
I'm so custom
</AccordionCard>
</Accordion>;
dangerouslySetHeader
allows greater flexibility with header content but we recommend that you do not overuse this property.
There are deprecated versions of this component. Switch to a version of the docs older than 10.0.x to view their docs.
Usage
Overview
The accordion is a component that allows for progressive display of information. Accordions are useful when you need to deliver large amounts of content within a small space (for example, mobile interface or side panel). They consist of vertically stacked headers, which expand to reveal their associated content. Accordions give users control over how much information they see on the screen at any given time. You might encounter accordions in wizard flows and in mobile solutions, where space is at a premium.
When to use
- To disclose content that users can selectively read.
- When there isn't a lot of vertical space and the content doesn't all need to be displayed at once.
- To organize related information.
When not to use
- For content that is critical to task completion.
- When you're creating a menu. Instead, consider using the overflow menu component.
- When you need to nest multiple accordions inside each other. Instead, consider using the tree view component.
Anatomy
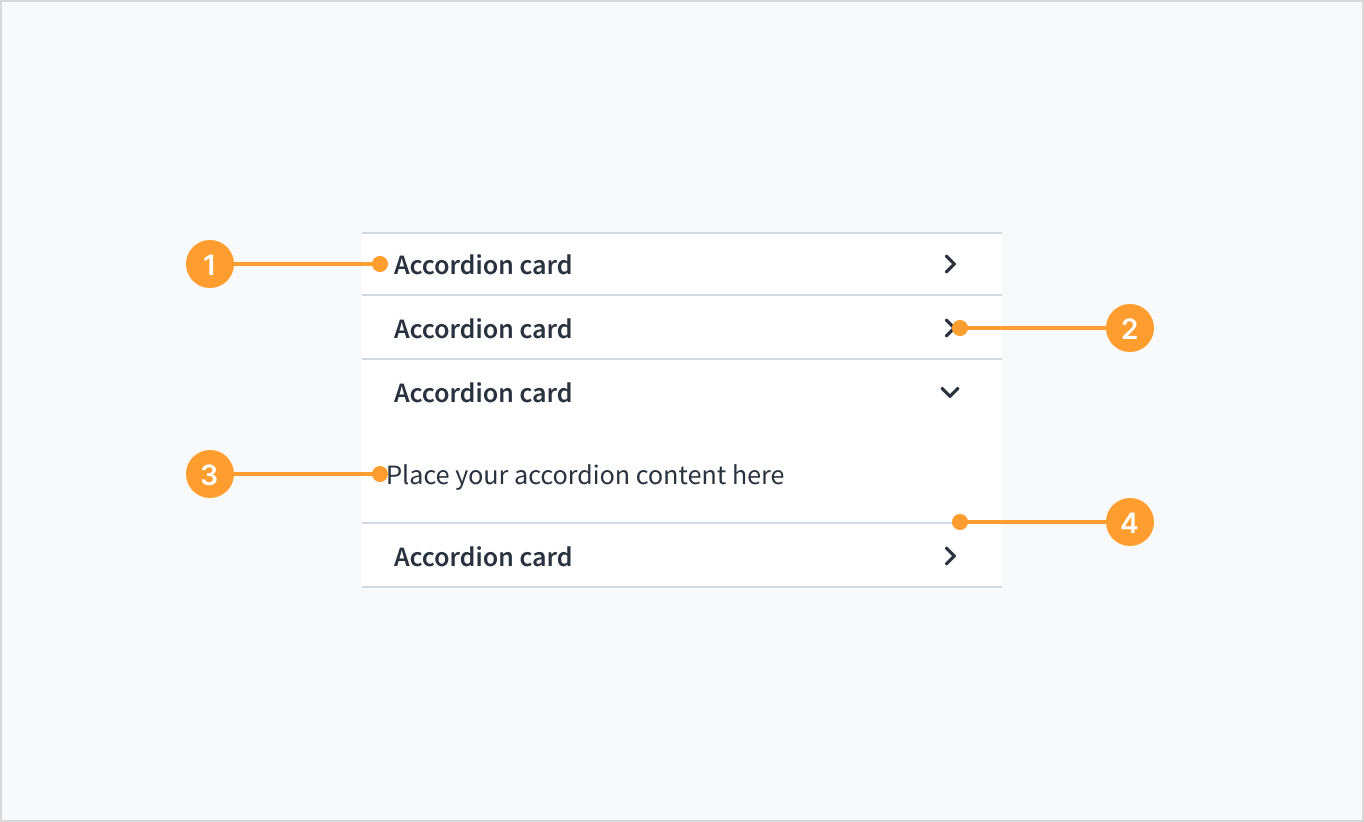
The accordion consists of the following elements:
- Header: Title that gives users an overview of the content in that section.
- Chevron: Icon that indicates the status of the accordion (expanded or collapsed).
- Main content: Associated content that appears when expanding the accordion header. May consist of text or UI elements such as an input field.
- Divider: Visual delimiter to distinguish accordion items. It should be visible by default with the option to hide it.
Alignment and placement
By default, the chevron icon is placed on the right side (at the end) of the header. This allows for the accordion title to align with other content on the page. Icon placement (left or right) must be consistent across all pages within one app.
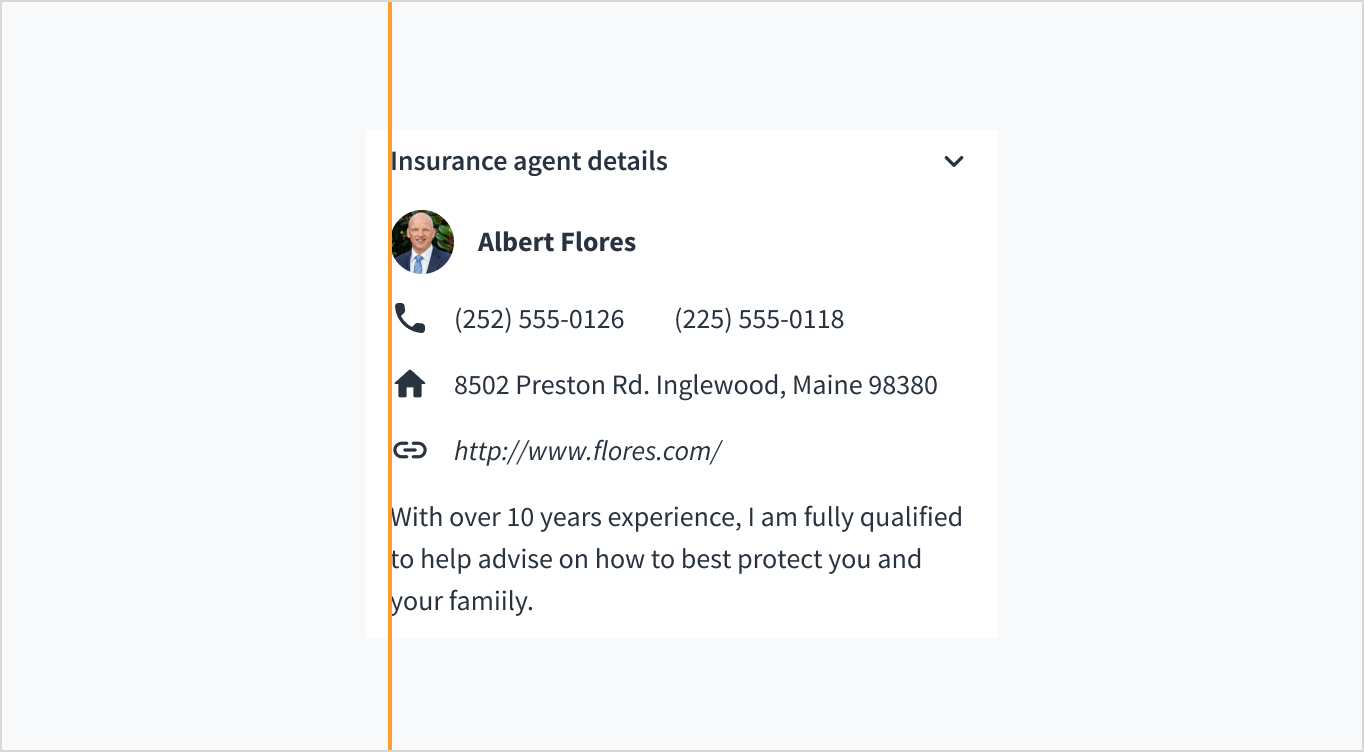
The accordion must fit the whole width of the parent container. To change the width of the accordion, change the container width.
Do match the width of the accordion to the width of the parent container.
Don't make the width of the accordion less than the width of the parent container.
Content
General writing guidelines
- Use sentence case for all aspects of designing Guidewire product interfaces. Don't use title case.
- Use present tense verbs and active voice in most situations.
- Use common contractions to lend your copy a more natural and informal tone.
- Use plain language. Avoid unnecessary jargon and complex language.
- Keep words and sentences short.
The accordion header can contain the following:
- Text (header title and secondary text).
- Icon (When the chevron is on the right side, you can place an icon before the header title. If the chevron is on the left side, place the icon at the end of the accordion header).
The accordion header can include text, icons, and other non-interactive elements.
Don't add interactive elements, such as buttons, to the accordion header. This violates accessibility.
Each accordion header must have a unique title that clearly and succinctly describes the associated body content. Headers appear in sentence case, except when using proper nouns.
Accordion body
- Content in accordions should never scroll horizontally.
- You may split the contents of an accordion panel into paragraphs and include sub-headers if needed.
Word, character limits
There is no maximum character or word limit on the header or the main content.
Behaviors
States
The accordion component has 2 main states: collapsed and expanded.
- Collapsed: Default state when the accordion is collapsed and not selected. Closed accordions appear without highlighting and display only the header title.
- Expanded: Indicates that the user has selected the accordion and expanded it to display content. The chevron icon points downwards to indicate that the accordion has been expanded.
In addition to the collapsed and expanded states, accordions also have interactive states for enabled, disabled, hover, focus, and active.
| State | Description |
---|
 | Enabled | Default state. |
 | Disabled | Indicates that the accordion is not interactive but might be at some point. Disabled state is not meant to be accessible so it should not be focused. |
 | Hover | Indicates a change of styling when the user hovers over the accordion header using a mouse. |
 | Focus | Indicates that the user has selected (but not expanded) the accordion. The borders of the component are highlighted with our brand color. |
 | Active | Indicates that the user is clicking or tapping the element. |
Interactions
Mouse
When a user clicks to open a new accordion item, the items that are already opened remain so. Each interaction requires a user click to prevent disorientation in the UI. Users can collapse and expand the accordion by clicking on the chevron or by clicking anywhere in the header region.
Keyboard
The accordion header functions as a button. Enter and Space expand and collapse each accordion panel. Navigate through the focusable accordion elements using Tab and Shift+Tab.
Screen reader
When collapsed, accordion panel content is hidden. The WAI-ARIA state aria-expanded="false"
advises users that the panel isn't visible. When expanded, the ARIA attribute changes to 'true' to convey that the content is visible.
Accessibility
The contrast ratio of elements is above 4.5:1, as per WCAG 2.0 AA requirements. All content is visible and functional up to and including a zoom factor of 200%.
This component has been validated to meet the WCAG 2.0 AA accessibility guidelines. However, changes made by the content author can affect accessibility conformance. Follow the guidance below and also refer to the WAI-ARIA Authoring Practices for Accordions when using this component in your applications:
- Each accordion header must have a unique title that describes the accordion panel content. This assists blind users, in particular, in that they can navigate through each header and understand the content, without having to expand each panel.
- Ensure that there are no keyboard traps when adding content to the accordion panel. Users should be able to move to each focusable element within each panel and then move to the next accordion card header.
There are deprecated versions of this component. Switch to a version of the docs older than 10.0.x to view their docs.
Code
<Accordion>
<AccordionCard title="Card 1">Test card</AccordionCard>
<AccordionCard title="Card 2">Test card 2</AccordionCard>
<AccordionCard
disabled
title="Card 3">
Test card 3
</AccordionCard>
</Accordion>
Import statement
import { Accordion, AccordionCard } from '@jutro/components';
Component contract
Make sure to understand the Design System components API surface, and the implications and trade-offs. Learn more in our introduction to the component API.
Properties
Accordion
children
required
DescriptionAccepts one or many AccordionCards that will be rendered in context of this Accordion container
chevronPosition
DescriptionAllows to change the position of the chevron icon for all the AccordionCards in the container
className
DescriptionAdditional className that will be passed to the root of the Accordion component
hideDivider
DescriptionIf true, the dividers between AccordionCards in the container will be hidden
AccordionCard
className
DescriptionAdditional className that will be passed to the root of the AccordionCard component
disabled
DescriptionIf true, the entire AccordionCard component will be disabled and proper styles will be applied
initialExpanded
DescriptionIf true, the AccordionCard will be expanded on initial load
Passing HTML properties to the AccordionCard
component
Under the hood, AccordionCard
renders a native HTML Button element. If there is any HTML property from the standard HTML Button that is not already part of the AccordionCard
component, you can pass it to the component. It will be assigned to the internal HTML Button component.
<AccordionCard
title="Card title"
id="accordion-card-1"
/>
The result of passing this id
property is that it will be part of the HTML button that is created in the HTML DOM.
Hooks
useAccordionCardState
- Provides props from the AccordionCard
context that you can use to create custom AccordionCard
headers. The hook returns a value, which is an object that contains:
disabled
required
expanded
required
title
const CustomHeader = () => {
const { expanded, disabled, title } = useAccordionCardState();
return (...);
};
Translation keys
There are no translations for Accordion.
For information on how to manage translations, see our section about Internationalization.
Escape Hatches
For more information, see our documentation about escape hatches.
Are you using the legacy accordion?
- To view docs for the old components, switch to a version of the documentation older than 10.0.
- These legacy components are also available in Storybook:
Changelog
10.0.0
New @jutro/components/Accordion
component introduced replacing the previous one.
The Previous accordion-related components were deprecated and moved to the @jutro/legacy
package. To view their documentation, switch to version of the documentation older than 10.0.
Component | Old location | New location |
---|
Accordion | @jutro/components/Accordion | @jutro/legacy/components/Accordion |
AccordionCard | @jutro/components/AccordionCard | @jutro/legacy/components/AccordionCard |
Codemods are available to automatically rename existing imports.